by marycamcon24
Dynamic Status Display Enhancer
import logging
from gunicorn.app.base import BaseApplication
from app_init import create_initialized_flask_app
# Flask app creation should be done by create_initialized_flask_app to avoid circular dependency problems.
app = create_initialized_flask_app()
# Setup logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
class StandaloneApplication(BaseApplication):
def __init__(self, app, options=None):
self.application = app
self.options = options or {}
super().__init__()
def load_config(self):
# Apply configuration to Gunicorn
for key, value in self.options.items():
if key in self.cfg.settings and value is not None:
self.cfg.set(key.lower(), value)
def load(self):
Frequently Asked Questions
How can the Dynamic Status Display Enhancer benefit my e-commerce business?
The Dynamic Status Display Enhancer can significantly improve your e-commerce operations by providing real-time visibility into order statuses. This template allows you to quickly view and update order statuses, which can lead to improved customer satisfaction and more efficient order processing. The color-coded status indicators make it easy for your team to identify and prioritize orders at a glance, potentially reducing processing times and minimizing errors.
Can the Dynamic Status Display Enhancer be customized for different types of statuses beyond just orders?
Absolutely! While the template is initially set up for order statuses, it can be easily adapted for various business needs. For example, you could use it to track project statuses in a project management system, monitor inventory levels in a warehouse, or display the status of support tickets in a customer service dashboard. The flexible nature of the Dynamic Status Display Enhancer allows for easy customization to suit your specific business requirements.
How does the Dynamic Status Display Enhancer handle real-time updates?
The Dynamic Status Display Enhancer uses Vue.js to manage the front-end state and update the UI in real-time. When a status is changed, the updateOrderStatus
method is called, which can be connected to an API endpoint to persist the changes. Here's an example of how you might implement this method:
javascript
methods: {
updateOrderStatus(order) {
axios.put(`/api/orders/${order.id}/status`, { status: order.status })
.then(response => {
console.log('Order status updated:', order.id, order.status);
})
.catch(error => {
console.error('Error updating order status:', error);
});
}
}
This code assumes you're using Axios for API calls. You'd need to implement the corresponding API endpoint in your Flask backend to handle the status update.
Is it possible to integrate the Dynamic Status Display Enhancer with external systems or APIs?
Yes, the Dynamic Status Display Enhancer is designed with integration in mind. You can easily modify the template to fetch data from external APIs or push updates to other systems. This makes it versatile for businesses that need to synchronize status information across multiple platforms or services.
How can I add more status types to the Dynamic Status Display Enhancer?
Adding more status types is straightforward. You'll need to update both the front-end and back-end components. Here's an example of how you might modify the status-display
component to include a new "Shipped" status:
javascript
Vue.component('status-display', {
props: ['status'],
computed: {
statusName() {
switch (parseInt(this.status)) {
case 1: return 'Active';
case 2: return 'Inactive';
case 3: return 'Pending';
case 4: return 'Shipped'; // New status
default: return 'Unknown';
}
},
statusColor() {
switch (parseInt(this.status)) {
case 1: return 'bg-green-500';
case 2: return 'bg-red-500';
case 3: return 'bg-yellow-500';
case 4: return 'bg-blue-500'; // New status color
default: return 'bg-gray-500';
}
}
},
// ... rest of the component code
});
You would also need to update the select options in the HTML template and modify the backend model and database schema to accommodate the new status type. This flexibility allows you to tailor the Dynamic Status Display Enhancer to your specific business needs.
Created: | Last Updated:
Here's a step-by-step guide for using the Dynamic Status Display Enhancer template:
Introduction
The Dynamic Status Display Enhancer is a powerful template that allows you to create a real-time status display application. This app features a dashboard with color-coded status indicators that can be updated dynamically based on user input or API integration.
Getting Started
To begin using this template:
- Click the "Start with this Template" button in the Lazy Builder interface.
Test the Application
Once you've started with the template:
- Click the "Test" button in the Lazy Builder interface.
- This will initiate the deployment process and launch the Lazy CLI.
Using the Application
After the deployment is complete, you'll be provided with a link to access the application. The main features of the app include:
- A dashboard displaying order information including Order ID, Customer, Total, and Status.
- Color-coded status indicators (Active: Green, Inactive: Red, Pending: Yellow).
- A dropdown menu to update the status of each order.
To use the application:
- Open the provided link in your web browser.
- You'll see a table with sample order data.
- To change an order's status:
- Locate the order you want to update.
- Use the dropdown menu in the "Actions" column to select a new status.
- The status will update automatically, and the color-coded indicator will change accordingly.
Customizing the Application
To customize the application for your specific needs:
- Modify the
orders
array in thehome.js
file to include your actual order data. - Update the
updateOrderStatus
method inhome.js
to implement the API call for updating order status in your backend system. - Adjust the styling in
styles.css
to match your brand colors and preferences.
Integrating with a Backend API
To fully utilize this template, you should integrate it with a backend API that manages your order data. Here's a basic outline of how to do this:
- Update the
get_orders
route inroutes.py
to fetch real data from your database:
python
@app.route("/api/orders")
def get_orders():
orders = Order.query.all()
return jsonify([order.to_dict() for order in orders])
- Implement the
update_order_status
route inroutes.py
to handle status updates:
python
@app.route("/api/orders/<int:order_id>/status", methods=["PUT"])
def update_order_status(order_id):
new_status = request.json.get('status')
order = Order.query.get(order_id)
if order:
order.status = new_status
db.session.commit()
return jsonify({"message": "Order status updated successfully"}), 200
return jsonify({"message": "Order not found"}), 404
- Update the
updateOrderStatus
method inhome.js
to make an API call:
javascript
updateOrderStatus(order) {
fetch(`/api/orders/${order.id}/status`, {
method: 'PUT',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({ status: order.status }),
})
.then(response => response.json())
.then(data => console.log(data.message))
.catch(error => console.error('Error:', error));
}
By following these steps, you'll have a fully functional Dynamic Status Display Enhancer that can be easily integrated with your existing systems.
Template Benefits
-
Real-Time Order Management: This template provides a dynamic dashboard for administrators to monitor and update order statuses in real-time, improving operational efficiency and customer service.
-
Responsive Design: With both mobile and desktop layouts, the template ensures accessibility across various devices, allowing managers to stay updated on-the-go or from their desks.
-
Visual Status Indicators: The color-coded status display component enhances quick decision-making by providing instant visual cues about order statuses, reducing errors and improving response times.
-
Scalable Architecture: The use of Vue.js for frontend and Flask for backend, along with a modular structure, allows for easy scaling and addition of new features as the business grows.
-
Data-Driven Insights: By centralizing order data and statuses, this template lays the groundwork for generating valuable business insights, such as order processing times and status distribution, which can inform strategic decisions.
Technologies
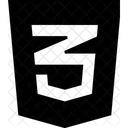
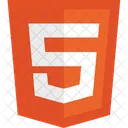