by davi
Discord Bot
import os
import discord
from discord import app_commands
from abilities import apply_sqlite_migrations
from models import Base, engine
def generate_oauth_link(client_id):
base_url = "https://discord.com/api/oauth2/authorize"
redirect_uri = "http://localhost"
scope = "bot"
permissions = "8" # Administrator permission for simplicity, adjust as needed.
return f"{base_url}?client_id={client_id}&permissions={permissions}&scope={scope}"
intents = discord.Intents.default()
intents.messages = True
intents.message_content = True
bot = discord.Client(intents=intents, activity=discord.CustomActivity("Example Activity"))
tree = app_commands.CommandTree(bot)
@bot.event
async def on_ready():
Frequently Asked Questions
What are some potential business applications for this Discord Bot template?
This Discord Bot template offers numerous business applications. It can be used to create customer support bots, automate community management tasks, build engagement tools for online communities, or develop internal communication bots for companies using Discord. The inclusion of a database makes it perfect for applications that require data persistence, such as user tracking, inventory management, or event scheduling.
How can this Discord Bot template improve user engagement in a business context?
The Discord Bot template can significantly enhance user engagement by providing interactive and automated responses. Businesses can use it to create custom commands for frequently asked questions, automate welcome messages for new members, or even implement gamification elements. The bot's ability to interact with a database allows for personalized experiences, such as tracking user activity or managing loyalty programs, which can greatly boost engagement and retention.
What industries could benefit most from implementing this Discord Bot template?
Several industries can benefit from this Discord Bot template. Gaming companies can use it to manage communities and provide game-related information. E-commerce businesses can implement it for customer service and order tracking. Educational institutions can utilize it for student support and course management. Tech companies can employ it for developer community management. The template's flexibility and database integration make it adaptable to various industry needs.
How can I add a custom command to the Discord Bot template?
To add a custom command to the Discord Bot template, you can use the @tree.command
decorator. Here's an example of how to add a simple "greet" command:
```python @tree.command(name='greet') async def greet_command(interaction, name: str): """Greet a user
Parameters
----------
name : str
The name of the user to greet (required)"""
await interaction.response.send_message(f"Hello, {name}! Welcome to our server!")
```
Add this code to the main.py
file. This command will allow users to use /greet
followed by a name, and the bot will respond with a greeting.
How can I interact with the database in this Discord Bot template?
The Discord Bot template uses SQLAlchemy for database interactions. To interact with the database, you first need to define your models in the models.py
file. Then, you can use SQLAlchemy's ORM to perform database operations. Here's a simple example:
```python from sqlalchemy import Column, Integer, String from models import Base, engine from sqlalchemy.orm import sessionmaker
# In models.py class User(Base): tablename = 'users' id = Column(Integer, primary_key=True) name = Column(String)
# In your command function Session = sessionmaker(bind=engine) session = Session()
new_user = User(name="John Doe") session.add(new_user) session.commit() ```
This example demonstrates how to define a model and add a new user to the database. Remember to create appropriate migrations in the migrations
folder for any model changes.
Created: | Last Updated:
Here's a step-by-step guide for using the Discord Bot template:
Introduction
This Discord Bot template provides a solid foundation for creating a custom bot with database integration. It includes basic command functionality and SQLite database support, making it an excellent starting point for various Discord bot projects.
Getting Started
- Click "Start with this Template" to begin working with this Discord Bot template in the Lazy Builder interface.
Initial Setup
Before running the bot, you'll need to set up a Discord application and obtain the necessary credentials. Follow these steps:
- Go to the Discord Developer Portal.
- Click "New Application" and give your application a name.
- Navigate to the "Bot" section in the left sidebar.
- Click "Add Bot" and confirm by clicking "Yes, do it!".
- Under the "TOKEN" section, click "Copy" to get your BOT_TOKEN.
- Go to the "OAuth2" section and copy your CLIENT_ID.
- In the Lazy Builder, open the Environment Secrets tab.
- Add two new secrets:
- Key:
CLIENT_ID
, Value: (paste your Client ID) - Key:
BOT_TOKEN
, Value: (paste your Bot Token) - In the Discord Developer Portal, under the "Bot" section, enable the "Message Content Intent" slider.
Test
Click the "Test" button in the Lazy Builder to start the deployment process.
Using the App
Once the bot is running, you'll see a message in the Lazy CLI with an OAuth link. Use this link to invite your bot to your Discord server:
🔗 Click this link to invite your Discord bot to your server 👉 [OAuth Link]
After inviting the bot to your server, you can use the /echo
command to test its functionality. The bot will repeat any text you provide after the command.
Integrating the App
To further customize your Discord bot:
- Modify the
main.py
file to add new commands or functionality. - Use the
models.py
file to define new database models if needed. - Create new migration files in the
migrations
folder to update your database schema.
Remember to redeploy your bot after making changes to see the updates in action.
By following these steps, you'll have a functional Discord bot with database support up and running, ready for further customization to meet your specific needs.
Template Benefits
-
Rapid Bot Development: This template provides a solid foundation for quickly developing Discord bots, allowing businesses to create custom solutions for community management, customer support, or internal communication without starting from scratch.
-
Database Integration: With built-in SQLAlchemy and migration support, businesses can easily store and manage data, enabling features like user tracking, analytics, or persistent settings for more sophisticated bot functionalities.
-
Scalability and Maintainability: The modular structure and use of modern Discord.py features (like app commands) make it easier to scale the bot's capabilities and maintain the codebase as the project grows, reducing long-term development costs.
-
Enhanced User Engagement: By leveraging Discord's rich features through this template, businesses can create interactive and engaging experiences for their community members, potentially increasing user retention and participation.
-
Customizable Automation: The template's flexibility allows businesses to automate various tasks within their Discord servers, such as moderation, event scheduling, or information dissemination, freeing up human resources for more complex tasks.
Technologies
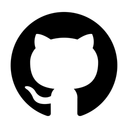

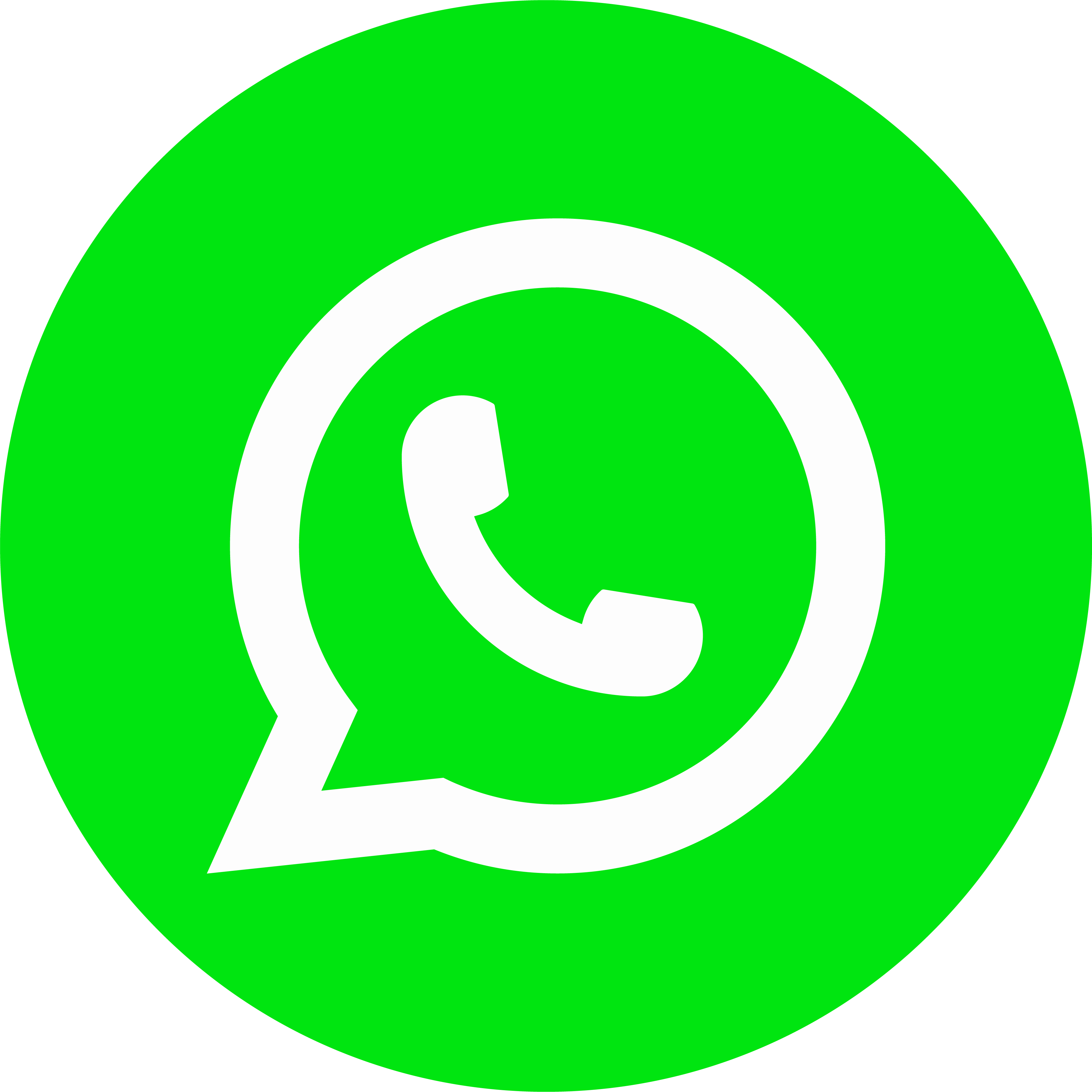
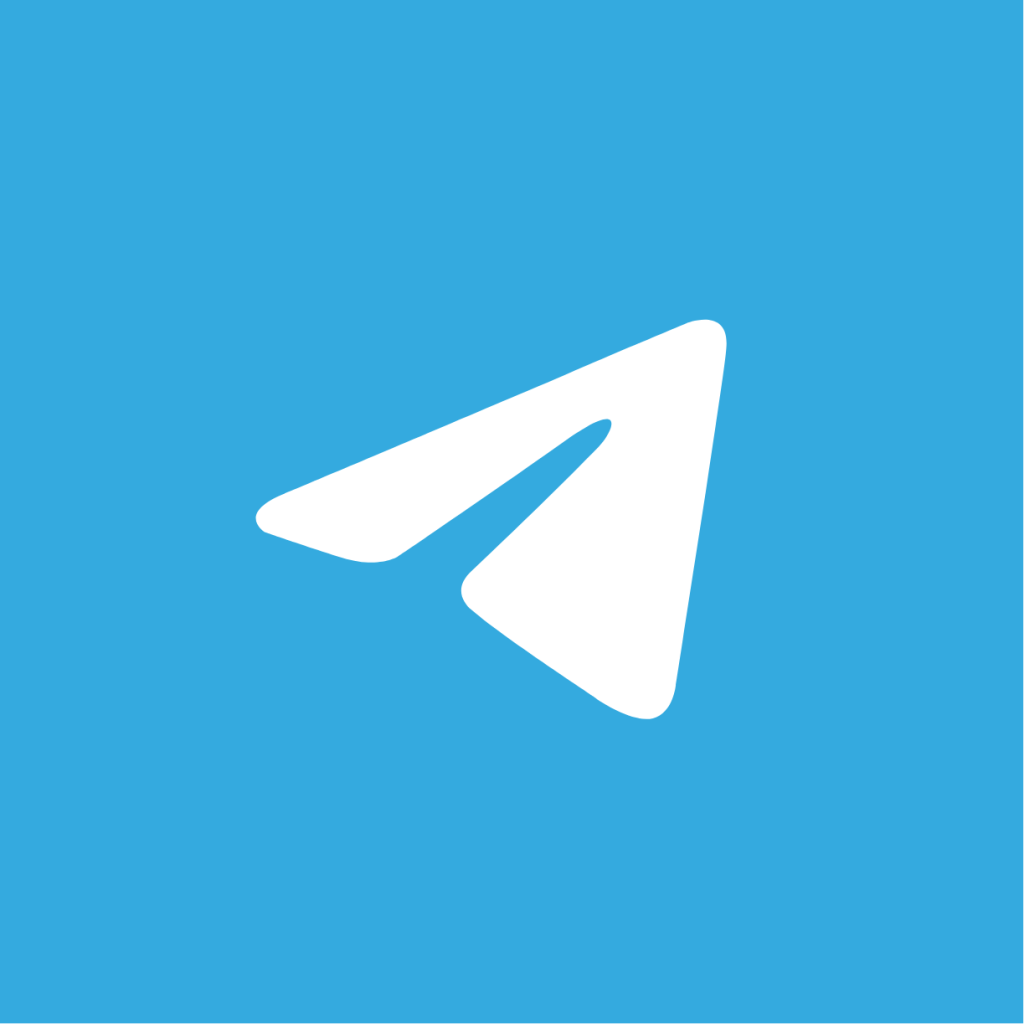
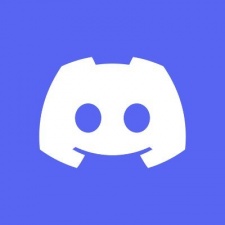