by ips
Basic Discord Bot
import os
import discord
from discord.ext import commands
def generate_oauth_link(client_id):
base_url = "https://discord.com/api/oauth2/authorize"
redirect_uri = "http://localhost"
scope = "bot"
permissions = "8" # Administrator permission for simplicity, adjust as needed.
return f"{base_url}?client_id={client_id}&permissions={permissions}&scope={scope}"
def start_bot(token):
intents = discord.Intents.default()
intents.messages = True
bot = commands.Bot(command_prefix='!', intents=intents)
@bot.event
async def on_ready():
print(f'Bot is ready. Logged in as {bot.user}')
bot.run(token)
def main():
client_id = os.environ.get('CLIENT_ID')
Frequently Asked Questions
What are some potential business applications for this Discord Bot template?
This Discord Bot template offers numerous business applications. It can be used to create customer support bots for handling inquiries in Discord communities, develop engagement tools for brand communities, automate moderation tasks in large servers, or even create interactive product guides and tutorials. The template's flexibility allows it to be adapted for various business needs within the Discord ecosystem.
How can this Discord Bot template improve community engagement?
The Discord Bot template provides a foundation for creating interactive and responsive bots that can significantly enhance community engagement. By implementing custom commands, automated responses, and interactive features, businesses can use this template to create bots that facilitate discussions, organize events, conduct polls, or provide real-time information to community members. This increased interactivity can lead to higher user retention and more active participation in Discord servers.
What industries could benefit most from implementing a bot based on this template?
Several industries can benefit from implementing a bot based on this Discord Bot template: - Gaming companies can use it to create game-specific bots for their communities - E-learning platforms can develop bots to assist students and manage course-related queries - Tech startups can create support bots to handle customer inquiries - Media companies can use bots to distribute content updates and engage with their audience - E-commerce businesses can develop bots for order tracking and customer service
How can I add a new command to the Discord Bot template?
To add a new command to the Discord Bot template, you can use the @tree.command
decorator. Here's an example of how to add a simple "greet" command:
```python @tree.command(name='greet') async def greet_command(interaction, name: str): """Greet a user
Parameters
----------
name : str
The name of the user to greet (required)"""
await interaction.response.send_message(f"Hello, {name}!")
```
Add this code to the main.py
file, and the bot will now respond to the /greet
command with a personalized greeting.
How can I modify the bot's activity status in this Discord Bot template?
The bot's activity status is set when initializing the discord.Client
object. To modify it, you can change the activity
parameter in the main.py
file. For example, to set the bot's status to "Listening to commands", you would modify the following line:
python
bot = discord.Client(intents=intents, activity=discord.Activity(type=discord.ActivityType.listening, name="commands"))
This change will update the bot's status to show "Listening to commands" in the Discord user list.
Created: | Last Updated:
Introduction to the Discord Bot Template
Welcome to the Discord Bot Template on Lazy! This template is designed to help you create a Discord bot with ease. The bot is capable of responding to commands and can be customized to suit your needs. Whether you're looking to automate tasks, moderate your server, or add fun interactions for your community, this template is a great starting point.
Getting Started with the Template
To begin using this template, simply click on "Start with this Template" on the Lazy platform. This will pre-populate the code in the Lazy Builder interface, so you won't need to copy, paste, or delete any code manually.
Initial Setup: Adding Environment Secrets
Before you can test and deploy your Discord bot, you'll need to set up a couple of environment secrets within the Lazy Builder. These secrets are the CLIENT_ID and BOT_TOKEN, which are essential for your bot to interact with the Discord API.
- Go to the Discord Developer Portal and create a new application.
- Navigate to the 'Bot' section and click 'Add Bot'.
- Under the 'TOKEN' section, click 'Copy' to get your BOT_TOKEN.
- Navigate to the 'OAuth2' section, and under 'CLIENT ID', click 'Copy' to get your CLIENT_ID.
- Back in the Lazy Builder, go to the Environment Secrets tab.
- Add two new secrets: one named CLIENT_ID and the other named BOT_TOKEN, pasting the respective values you copied from the Discord Developer Portal.
Test: Deploying the Bot
With your environment secrets in place, you're ready to deploy your bot. Press the "Test" button on the Lazy platform. This will begin the deployment of your app and launch the Lazy CLI. You won't need to provide any additional user input at this stage, as the CLIENT_ID and BOT_TOKEN are already set as environment secrets.
Using the Bot
Once your bot is deployed, you can invite it to your Discord server using the OAuth link that will be printed in the Lazy CLI. The bot will start automatically and will be ready to respond to commands in your server. You can interact with your bot by typing commands prefixed with '!' (or any other prefix you set) in your Discord server's text channels.
Integrating the Bot
To fully integrate your bot into your Discord server, you may want to customize its commands and functionality. Here's a sample of how you might add a simple command to your bot:
@bot.command()
async def greet(ctx):
await ctx.send("Hello, I'm your friendly Discord bot!")
Add this code snippet to your main.py file in the Lazy Builder interface to create a new command that makes your bot greet users when they type '!greet'.
Remember to adjust the bot's permissions and functionality according to your server's needs. You can do this by modifying the permissions parameter in the generate_oauth_link function or by adding more commands and event listeners to your bot's code.
Once you're happy with your bot, you can share the OAuth link with your server members or add it to your server's website to allow others to invite the bot to their servers as well.
That's it! You've successfully set up and deployed a Discord bot using the Lazy platform. Enjoy your new bot, and have fun engaging with your community!
Here are 5 key business benefits for this Discord bot template:
Template Benefits
-
Rapid Bot Development: This template provides a solid foundation for quickly creating custom Discord bots, allowing businesses to save time and resources in the initial setup phase.
-
Enhanced Customer Support: Companies can use this bot framework to build automated support systems within Discord, improving response times and reducing the workload on human support staff.
-
Improved Community Engagement: By customizing the bot's commands, businesses can create interactive experiences for their Discord communities, fostering user engagement and loyalty.
-
Streamlined Information Dissemination: The bot can be programmed to automatically share updates, announcements, or important information, ensuring all community members stay informed efficiently.
-
Data Collection and Analytics: With further customization, the bot can be used to collect valuable user data and insights from Discord interactions, helping businesses make data-driven decisions about their community management and marketing strategies.
Technologies
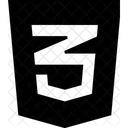
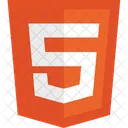
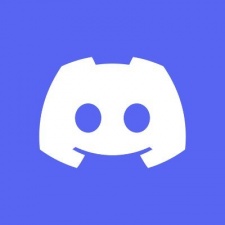