Crypto Alarm: Real-Time Price Tracker
import logging
from gunicorn.app.base import BaseApplication
from app_init import create_initialized_flask_app
# Setup logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
# Flask app creation should be done by create_initialized_flask_app to avoid circular dependency problems.
app = create_initialized_flask_app()
class StandaloneApplication(BaseApplication):
def __init__(self, app, options=None):
self.application = app
self.options = options or {}
super().__init__()
def load_config(self):
# Apply configuration to Gunicorn
for key, value in self.options.items():
if key in self.cfg.settings and value is not None:
self.cfg.set(key.lower(), value)
def load(self):
Frequently Asked Questions
How can Crypto Alarm benefit cryptocurrency traders and investors?
Crypto Alarm: Real-Time Price Tracker offers significant benefits for cryptocurrency traders and investors. It provides up-to-date price information for major cryptocurrencies like Bitcoin, Ethereum, BNB, XRP, and Cardano. This real-time data allows users to make informed decisions quickly, which is crucial in the fast-paced crypto market. Additionally, Crypto Alarm's alert functionality (which can be implemented as an extension) enables users to set custom price alerts, ensuring they never miss important market movements or potential trading opportunities.
Can Crypto Alarm be customized for specific business needs?
Yes, Crypto Alarm is highly customizable. Businesses can adapt the template to suit their specific requirements. For example, a cryptocurrency exchange could integrate Crypto Alarm into their platform to provide real-time price tracking for their users. Financial advisory firms could use it to monitor market trends and provide timely advice to clients. The template's modular structure allows for easy addition of new features or integration with existing systems, making it versatile for various business applications in the crypto space.
How does Crypto Alarm ensure data accuracy and reliability?
Crypto Alarm fetches its data from the CoinGecko API, a reputable source for cryptocurrency price information. The application is designed to update prices every 60 seconds, ensuring that users have access to the most current data. Here's a code snippet from the crypto.js
file that demonstrates this:
```javascript async function updateCryptoPrices() { const cryptoList = document.getElementById('crypto-list'); const data = await fetchCryptoPrices(); // ... update UI with new prices ... }
document.addEventListener('DOMContentLoaded', () => { // Initial load updateCryptoPrices();
// Auto refresh every 60 seconds
setInterval(updateCryptoPrices, 60000);
}); ```
This code ensures that Crypto Alarm regularly fetches and displays the latest price data, maintaining accuracy and reliability.
What potential revenue streams can be built around Crypto Alarm?
Crypto Alarm offers several potential revenue streams. Businesses could offer a freemium model where basic price tracking is free, but advanced features like customizable alerts, historical data analysis, or API access are part of a paid tier. Another option is to integrate affiliate links for cryptocurrency exchanges, earning commissions on user sign-ups or trades. Additionally, Crypto Alarm could be licensed to other businesses as a white-label solution, allowing them to offer branded price tracking services to their customers.
How can additional cryptocurrencies be added to Crypto Alarm's tracking list?
Adding more cryptocurrencies to Crypto Alarm is straightforward. You would need to modify the cryptoData
array in the crypto.js
file. Here's an example of how to add Dogecoin to the tracking list:
javascript
const cryptoData = [
// ... existing entries ...
{
id: 'dogecoin',
name: 'Dogecoin',
symbol: 'doge',
iconUrl: 'https://storage.googleapis.com/lazy-icons/crypto/dogecoin.png'
}
];
You would also need to update the API request in the fetchCryptoPrices
function to include the new cryptocurrency:
javascript
async function fetchCryptoPrices() {
try {
const response = await fetch('https://api.coingecko.com/api/v3/simple/price?ids=bitcoin,ethereum,binancecoin,ripple,cardano,dogecoin&vs_currencies=usd&include_24hr_change=true');
// ... rest of the function ...
}
}
These changes will seamlessly integrate the new cryptocurrency into Crypto Alarm's tracking and display system.
Created: | Last Updated:
Here's a step-by-step guide for using the Crypto Alarm: Real-Time Price Tracker template:
Introduction
The Crypto Alarm: Real-Time Price Tracker is a web application that provides real-time cryptocurrency price tracking. It displays current prices and 24-hour price changes for popular cryptocurrencies like Bitcoin, Ethereum, BNB, XRP, and Cardano.
Getting Started
- Click "Start with this Template" to begin using the Crypto Alarm template in the Lazy Builder interface.
Test the Application
-
Press the "Test" button in the Lazy Builder interface to deploy and launch the application.
-
Once the deployment is complete, you'll receive a dedicated server link to access the Crypto Price Tracker web interface.
Using the Crypto Price Tracker
-
Open the provided server link in your web browser to access the Crypto Price Tracker interface.
-
The main page displays a list of cryptocurrencies with their current prices and 24-hour price changes.
-
To refresh the prices manually, click the "Refresh Prices" button at the top of the page.
-
The prices are automatically updated every 60 seconds.
-
The "Last updated" timestamp at the top of the page shows when the prices were last refreshed.
Features
- Real-time price tracking for Bitcoin, Ethereum, BNB, XRP, and Cardano
- Displays current price in USD
- Shows 24-hour price change percentage
- Manual refresh option
- Automatic price updates every 60 seconds
- Responsive design for both desktop and mobile devices
Customization (Optional)
If you want to customize the tracked cryptocurrencies or add more features, you can modify the following files:
crypto.js
: Update thefetchCryptoPrices
function to include different cryptocurrencies or additional data.home.html
: Modify the HTML structure of the main page.styles.css
: Adjust the styling of the application.
Remember that any changes you make will require redeploying the application using the "Test" button in the Lazy Builder interface.
By following these steps, you'll have a fully functional Crypto Price Tracker application up and running, providing real-time cryptocurrency price information to your users.
Here are 5 key business benefits for the Crypto Price Tracker template:
Template Benefits
-
Real-Time Market Insights: Provides up-to-date cryptocurrency price information, enabling users to make informed investment decisions quickly.
-
Mobile-Responsive Design: Offers a seamless experience across desktop and mobile devices, allowing users to access critical market data on-the-go.
-
Easy Integration: Built with Flask and modern web technologies, making it simple to integrate into existing financial platforms or expand with additional features.
-
Customizable Alerts: Can be easily extended to include price alert functionality, helping users stay on top of market movements without constant monitoring.
-
Scalable Architecture: Utilizes Gunicorn for production deployment, ensuring the application can handle increased traffic as user base grows.
Technologies
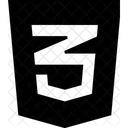
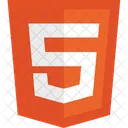
