by Lazy Sloth
Basic Discord Bot
import os
import discord
from discord.ext import commands
intents = discord.Intents.default()
# Initialize the bot with "/" as the prefix
bot = commands.Bot(command_prefix="/", intents=intents)
bot_token = os.environ.get('DISCORD_BOT_TOKEN')
# Event: Bot is ready
@bot.event
async def on_ready():
print(f'Logged in as {bot.user.name}')
def main():
# Checking if the Discord bot token exists
if not bot_token:
print("Let's fix that together! 😊 Here's how:\n" +
"1. 🌐 Go to the Discord Developer Portal. 🌐\n" +
"2. 🆕 Create a new application and navigate to the 'Bot' section. 🤖\n" +
"3. 📋 Under the 'TOKEN' section, click 'Reset Token' to get your Discord bot token and confirm by clicking 'Yes, do it'. 🔑\n" +
"4. 🤖 If your bot will have any ability to read/reply to messages, then under the 'BOT' section, enable the slider titled 'Message Content Intent' ✅" +
"5. 🔐 Set the 'DISCORD_BOT_TOKEN' in the Env Secrets tab with your bot token from step 3. 🔐\n" +
Frequently Asked Questions
What are some potential business applications for this Basic Discord Bot template?
The Basic Discord Bot template serves as an excellent starting point for various business applications. Some potential uses include: - Customer support chatbot for handling basic inquiries - Event announcement and reminder system for company Discord servers - Employee engagement tool for team-building activities or company updates - Social media content aggregator for marketing teams - Project management assistant for tracking tasks and deadlines
How can this Basic Discord Bot template improve communication within a company?
The Basic Discord Bot template can significantly enhance internal communication by: - Automating routine announcements and reminders - Providing a centralized platform for information dissemination - Facilitating quick polls or surveys for team decision-making - Offering an interactive FAQ system for common company policies or procedures - Streamlining onboarding processes for new employees
What industries could benefit most from implementing a bot based on this Basic Discord Bot template?
While the Basic Discord Bot template is versatile, some industries that could particularly benefit include: - Gaming companies for community management and support - E-commerce businesses for customer service and order tracking - Educational institutions for student engagement and information distribution - Tech startups for developer community management and support - Media companies for content distribution and audience interaction
How can I add a simple command to the Basic Discord Bot template?
To add a simple command to the Basic Discord Bot template, you can use the @bot.command()
decorator. Here's an example of adding a "hello" command:
python
@bot.command()
async def hello(ctx):
await ctx.send(f"Hello, {ctx.author.name}!")
Add this code to the main.py
file, and users can then use the command by typing "/hello" in the Discord chat.
How can I make the Basic Discord Bot respond to specific keywords in messages?
You can modify the on_message
event to check for specific keywords. Here's an example:
```python @bot.event async def on_message(message): if message.author == bot.user: return
if "help" in message.content.lower():
await message.channel.send("Need assistance? Here are some commands you can use...")
await bot.process_commands(message)
```
This code will make the Basic Discord Bot respond with a help message whenever the word "help" is mentioned in a message. Remember to keep the await bot.process_commands(message)
line to ensure other commands still work.
Created: | Last Updated:
Introduction to the Basic Discord Bot Template
Welcome to the Basic Discord Bot template! This template provides you with the foundational code to create your very own Discord bot. Whether you're looking to build a bot for fun, for your community, or for productivity, this template is the perfect starting point. It comes with the essential setup that allows your bot to log in and be ready for further development.
Getting Started with the Template
To begin using this template, simply click on "Start with this Template" on the Lazy platform. This will set up the template in your Lazy Builder interface, pre-populating the code so you can start customizing your bot right away.
Initial Setup: Adding Environment Secrets
Before you can test and deploy your bot, you'll need to set up an environment secret for your Discord bot token. Here's how to do it:
- Go to the Discord Developer Portal and create a new application.
- Navigate to the 'Bot' section and click 'Reset Token' to get your Discord bot token.
- Enable the 'Message Content Intent' if your bot will read or reply to messages.
- In the Lazy Builder, go to the Environment Secrets tab.
- Set the 'DISCORD_BOT_TOKEN' with the token you obtained from the Discord Developer Portal.
Remember, this token is like a password for your bot, so keep it secure and never share it publicly.
Test: Deploying the App
Once you have set up your environment secret, you can deploy your bot by pressing the "Test" button. This will launch the Lazy CLI, and if there are any additional prompts for user input, you will be able to provide it there.
Using the App
After deploying your bot, it will be live on Discord, but it won't do much yet. This is where your creativity comes in! You can start adding commands and functionalities to your bot by editing the code in the Lazy Builder interface. Use the provided template as a starting point to build the features you want.
Integrating the App
Once your bot is ready and has the functionalities you desire, you can invite it to your Discord server to start interacting with users. To do this, you'll need to generate an invite link from the Discord Developer Portal and use it to add your bot to the server.
- In the Discord Developer Portal, navigate to the 'OAuth2' section.
- Select the 'bot' scope and the permissions your bot requires.
- Use the generated URL to invite your bot to your Discord server.
Now your bot is integrated into your server and ready to interact with your community!
Remember, this is just the beginning. As you grow more comfortable with the code and the Lazy platform, you can continue to expand your bot's capabilities and make it truly unique. Happy building!
Template Benefits
-
Rapid Prototyping: This template provides a quick starting point for businesses to develop custom Discord bots, allowing for fast ideation and testing of new features without extensive setup.
-
Cost-Effective Development: By offering a basic, functional structure, this template reduces initial development time and costs, enabling businesses to allocate resources more efficiently.
-
Scalable Communication Tool: Businesses can easily expand this bot to create tailored communication channels with their Discord community, enhancing customer engagement and support.
-
Customizable Automation: The template serves as a foundation for automating various tasks within a Discord server, such as moderating content, managing roles, or distributing information, improving operational efficiency.
-
Integration Potential: This basic structure can be extended to integrate with other business systems or APIs, creating a powerful tool for data collection, analysis, and cross-platform interactions.
Technologies
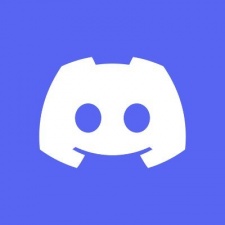