AI Chat
import logging
from gunicorn.app.base import BaseApplication
from app_init import create_initialized_flask_app
# Setup logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
# Flask app creation should be done by create_initialized_flask_app to avoid circular dependency problems.
app = create_initialized_flask_app()
class StandaloneApplication(BaseApplication):
def __init__(self, app, options=None):
self.application = app
self.options = options or {}
super().__init__()
def load_config(self):
# Apply configuration to Gunicorn
for key, value in self.options.items():
if key in self.cfg.settings and value is not None:
self.cfg.set(key.lower(), value)
def load(self):
Frequently Asked Questions
What are some potential business applications for the Simple AI Text Analyzer?
The Simple AI Text Analyzer has various business applications across different industries. Some potential use cases include: - Customer service: Analyzing customer feedback and support tickets to gauge sentiment and identify areas for improvement. - Market research: Evaluating social media posts and product reviews to understand public opinion about brands or products. - HR and employee engagement: Analyzing internal communications and employee surveys to assess workplace satisfaction and morale. - Content marketing: Evaluating the tone and sentiment of blog posts, articles, or social media content to ensure they align with brand voice and audience preferences.
How can the Simple AI Text Analyzer be customized for specific industry needs?
The Simple AI Text Analyzer can be tailored to meet specific industry requirements by: - Training the AI model on industry-specific datasets to improve accuracy and relevance. - Implementing custom sentiment categories or metrics relevant to the industry (e.g., technical jargon analysis for IT companies). - Integrating with industry-specific tools and platforms (e.g., CRM systems for sales and marketing teams). - Adding features like trend analysis or competitor comparison for more comprehensive insights.
What are the potential cost savings associated with implementing the Simple AI Text Analyzer in a business?
Implementing the Simple AI Text Analyzer can lead to significant cost savings for businesses by: - Reducing manual labor required for sentiment analysis and text processing. - Improving decision-making through data-driven insights, potentially avoiding costly mistakes. - Enhancing customer satisfaction and retention by quickly identifying and addressing issues. - Streamlining market research processes, reducing the need for expensive third-party services. - Optimizing content creation and marketing strategies based on sentiment analysis results.
How can I modify the chat route in the Simple AI Text Analyzer to include sentiment analysis?
To add sentiment analysis to the chat route, you can modify the chat
function in routes.py
. Here's an example of how you could implement this:
```python from textblob import TextBlob
@app.route("/chat", methods=["POST"]) def chat(): data = request.get_json() message = data.get("message", "")
# Perform sentiment analysis
blob = TextBlob(message)
sentiment = blob.sentiment.polarity
response_schema = {
"type": "object",
"properties": {
"response": {
"type": "string",
"description": "The chatbot's response"
},
"sentiment": {
"type": "number",
"description": "Sentiment score (-1 to 1)"
}
},
"required": ["response", "sentiment"]
}
try:
result = llm(
prompt=f"You are a friendly and helpful AI assistant. Respond to this message: {message}",
response_schema=response_schema,
image_url=None,
model="gpt-4o",
temperature=0.7
)
return jsonify({"response": result["response"], "sentiment": sentiment})
except Exception as e:
logger.error(f"Error in chat: {str(e)}")
return jsonify({"error": "Failed to process message"}), 500
```
This modification adds sentiment analysis using the TextBlob library and includes the sentiment score in the response.
How can I extend the Simple AI Text Analyzer to handle multiple languages?
To extend the Simple AI Text Analyzer for multiple languages, you can use a language detection library and adjust the sentiment analysis accordingly. Here's an example of how you could modify the chat
function to support multiple languages:
```python from textblob import TextBlob from langdetect import detect
@app.route("/chat", methods=["POST"]) def chat(): data = request.get_json() message = data.get("message", "")
# Detect language
try:
language = detect(message)
except:
language = "en" # Default to English if detection fails
# Perform sentiment analysis based on language
if language == "en":
blob = TextBlob(message)
sentiment = blob.sentiment.polarity
else:
# Use a different sentiment analysis method for non-English text
# This is a placeholder and should be replaced with an appropriate method
sentiment = 0
response_schema = {
"type": "object",
"properties": {
"response": {
"type": "string",
"description": "The chatbot's response"
},
"sentiment": {
"type": "number",
"description": "Sentiment score (-1 to 1)"
},
"language": {
"type": "string",
"description": "Detected language code"
}
},
"required": ["response", "sentiment", "language"]
}
try:
result = llm(
prompt=f"You are a friendly and helpful AI assistant. Respond to this message in the same language: {message}",
response_schema=response_schema,
image_url=None,
model="gpt-4o",
temperature=0.7
)
return jsonify({"response": result["response"], "sentiment": sentiment, "language": language})
except Exception as e:
logger.error(f"Error in chat: {str(e)}")
return jsonify({"error": "Failed to process message"}), 500
```
This modification adds language detection and adjusts the sentiment analysis based on the detected language. Note that you'll need to implement or integrate appropriate sentiment analysis methods for non-English languages to make this fully functional.
Created: | Last Updated:
Here's a step-by-step guide for using the Simple AI Text Analyzer template:
Introduction
The Simple AI Text Analyzer is a web-based tool that provides sentiment insights using AI. This template sets up a Flask application with a chat interface, allowing users to input text and receive AI-generated responses.
Getting Started
- Click "Start with this Template" to begin using the Simple AI Text Analyzer template in the Lazy Builder interface.
Test the Application
- Press the "Test" button in the Lazy Builder interface to start the deployment process and launch the Lazy CLI.
Using the Application
-
Once the deployment is complete, Lazy will provide you with a dedicated server link to access the web interface.
-
Open the provided link in your web browser to access the Simple AI Text Analyzer interface.
-
You'll see a chat interface with an input box at the bottom.
-
Type your message or the text you want to analyze in the input box.
-
Press the "Send" button or hit Enter to submit your message.
-
The AI will process your input and provide a response, which will appear in the chat interface.
How It Works
- The application uses a language model (LLM) to generate responses based on user input.
- The AI is configured to act as a friendly and helpful assistant, providing insights and analysis of the text you submit.
- The chat interface allows for continuous interaction, so you can ask follow-up questions or submit multiple texts for analysis.
Additional Notes
- The application is designed to be user-friendly and doesn't require any additional setup or integration steps.
- All processing occurs on the server-side, so you don't need to worry about local resources or installations.
- The chat history is maintained within the current session, allowing for contextual conversations.
By following these steps, you'll have a fully functional AI Text Analyzer running on the Lazy platform, ready to provide sentiment insights and engage in helpful conversations based on the text you input.
Here are 5 key business benefits for this AI chatbot template:
Template Benefits
-
Rapid Deployment: This template provides a fully functional AI chatbot interface that can be quickly deployed, allowing businesses to implement conversational AI solutions with minimal setup time.
-
Scalable Architecture: Built with Flask and Gunicorn, the template offers a scalable foundation that can handle increasing user loads, making it suitable for growing businesses.
-
Responsive Design: The template includes both mobile and desktop layouts, ensuring a seamless user experience across different devices, which is crucial for maximizing user engagement.
-
Customizable AI Integration: The template is set up to easily integrate with various AI models (currently using GPT-4), allowing businesses to tailor the chatbot's capabilities to their specific needs.
-
Modern UI/UX: With a clean, modern interface and real-time chat functionality, this template provides an engaging user experience that can help businesses improve customer interactions and satisfaction.
Technologies
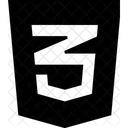
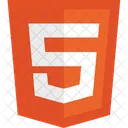