3D SBS Video Converter
import logging
from gunicorn.app.base import BaseApplication
from app_init import create_initialized_flask_app
# Flask app creation should be done by create_initialized_flask_app to avoid circular dependency problems.
app = create_initialized_flask_app()
# Setup logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
class StandaloneApplication(BaseApplication):
def __init__(self, app, options=None):
self.application = app
self.options = options or {}
super().__init__()
def load_config(self):
# Apply configuration to Gunicorn
for key, value in self.options.items():
if key in self.cfg.settings and value is not None:
self.cfg.set(key.lower(), value)
def load(self):
Frequently Asked Questions
What is the main purpose of the 3D SBS Video Converter application?
The 3D SBS Video Converter is designed to provide a user-friendly interface for uploading and potentially converting 3D side-by-side (SBS) video files. It allows users to upload video files in formats such as MP4, MOV, and AVI through a web interface. While the current template focuses on the upload functionality, it serves as a foundation for implementing 3D SBS video conversion features in future iterations.
How can businesses benefit from integrating the 3D SBS Video Converter into their workflow?
Businesses in the entertainment, gaming, or virtual reality industries can greatly benefit from the 3D SBS Video Converter. It can streamline the process of handling 3D content by providing a centralized platform for uploading and managing 3D videos. This tool can be particularly useful for content creators, film studios, or VR developers who frequently work with 3D video formats and need an efficient way to process or distribute their content.
Is it possible to customize the 3D SBS Video Converter for specific business needs?
Absolutely! The 3D SBS Video Converter template is built with Flask, a flexible Python web framework, making it highly customizable. Businesses can easily extend the functionality to include specific features such as batch processing, integration with existing content management systems, or adding proprietary 3D conversion algorithms. The modular structure of the application allows for seamless additions and modifications to suit various business requirements.
How can I add a new route to handle video conversion in the 3D SBS Video Converter?
To add a new route for video conversion, you can modify the routes.py
file. Here's an example of how you might add a conversion route:
```python @app.route("/convert", methods=["POST"]) def convert_video(): if "video" not in request.files: flash("No file part") return redirect(url_for("home_route")) file = request.files["video"] if file and allowed_file(file.filename): filename = secure_filename(file.filename) input_path = os.path.join(app.config["UPLOAD_FOLDER"], filename) file.save(input_path) output_path = os.path.join(app.config["UPLOAD_FOLDER"], "converted_" + filename)
# Call your conversion function here
# convert_to_3d_sbs(input_path, output_path)
flash("File converted successfully")
video_url = url_for('uploaded_file', filename="converted_" + filename)
return render_template("home.html", video_url=video_url)
else:
flash("Invalid file type")
return redirect(url_for("home_route"))
```
Remember to implement the actual conversion function (convert_to_3d_sbs
) and update the HTML template to include a conversion button that posts to this new route.
How can I modify the database schema in the 3D SBS Video Converter to store information about converted videos?
To store information about converted videos, you can create a new model in the database.py
file and add a corresponding migration. Here's an example:
Created: | Last Updated:
Here's a step-by-step guide for using the 3D SBS Video Converter template:
Introduction
The 3D SBS Video Converter template provides a web interface for uploading and converting 3D side-by-side video files. This template sets up a Flask-based web application with a simple user interface for file uploads and video playback.
Getting Started
To begin using this template:
- Click the "Start with this Template" button in the Lazy Builder interface.
Test the Application
Once you've started with the template:
- Click the "Test" button in the Lazy Builder interface.
- Wait for the application to deploy and start.
Using the 3D SBS Video Converter
After the application has started:
- Lazy will provide you with a dedicated server link to access the web interface.
- Open the provided link in your web browser.
- You'll see the 3D SBS Video Converter interface with an upload form.
To use the converter:
- Click the "Choose File" button to select a 3D side-by-side video file from your device.
- Supported file formats are MP4, MOV, and AVI.
- Once you've selected a file, click the "Upload" button.
- The file will be uploaded to the server.
- After a successful upload, you'll see a confirmation message.
- The uploaded video will be displayed on the page for playback.
Notes
- The application currently only handles file uploads and playback. It does not perform any actual 3D SBS video conversion.
- Uploaded files are stored in the
uploads
directory on the server. - The maximum file size for uploads is determined by your server configuration.
By following these steps, you'll have a functioning 3D SBS Video Converter web application up and running on the Lazy platform. You can further customize the template to add actual video conversion functionality or other features as needed.
Here are 5 key business benefits for the 3D SBS Video Converter template:
Template Benefits
-
Streamlined 3D Video Processing: Enables businesses to easily convert and process 3D side-by-side video files, saving time and resources in video production workflows.
-
User-Friendly Interface: Provides a simple, intuitive web interface for file uploads, making it accessible to both technical and non-technical users within an organization.
-
Scalable Architecture: Built with Flask and Gunicorn, the template offers a scalable foundation that can handle increased load as business needs grow.
-
Cross-Platform Compatibility: The web-based nature of the application ensures it can be accessed from various devices and operating systems, increasing flexibility for remote teams.
-
Customizable Framework: The modular structure of the template allows for easy customization and expansion, enabling businesses to add features specific to their 3D video processing needs.
Technologies
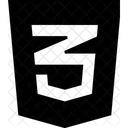
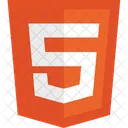