by coolzappmn
Zap Utilities
import os
import discord
import random
from discord import app_commands, Embed
from abilities import apply_sqlite_migrations, llm
from models import Base, engine
def generate_oauth_link(client_id):
base_url = "https://discord.com/api/oauth2/authorize"
redirect_uri = "http://localhost"
scope = "bot"
permissions = "8" # Administrator permission for simplicity, adjust as needed.
return f"{base_url}?client_id={client_id}&permissions={permissions}&scope={scope}"
def generate_server_structure(categories_count, channels_per_category, roles_count):
# Use LLM to generate Roblox-themed server structure with cool fonts
prompt = f"""Generate a Discord server structure for a Roblox gaming community with:
- {categories_count} categories
- {channels_per_category} channels per category
- {roles_count} roles
Make it Roblox-themed with appropriate emojis. Each category should have a theme, and channels should relate to that theme.
Use creative fonts and styles for names to make them look cool and unique."""
Created: | Last Updated:
Introduction
This template creates a Discord bot specifically designed for Roblox communities. The bot includes features like welcome/leave messages, moderation tools, and Roblox account information lookup capabilities.
Getting Started
- Click "Start with this Template" to begin using this template in Lazy Builder
Environment Setup
You'll need to set up one environment secret:
* BOT_TOKEN
: Your Discord bot token
To get your Discord bot token: * Go to the Discord Developer Portal * Create a new application or select an existing one * Go to the "Bot" section * Click "Reset Token" to reveal your bot token * Copy the token and add it to your Environment Secrets in Lazy Builder
Test the Bot
- Click the "Test" button to deploy your Discord bot
- The bot will start and connect to Discord using your provided token
Using the Bot
Once the bot is running, you can use these commands in your Discord server:
/set-welcome-channel
- Set up a channel for welcome messages/set-leave-channel
- Set up a channel for leave messages/gen-rblx-user
- Generate Roblox username suggestions/gen-rblx-avatar
- Get avatar style suggestions based on Robux budget/roblox-account-info [username]
- Look up detailed information about a Roblox account/deletecategory
- Delete an entire category and its channels (admin only)
Integrating with Discord
To add the bot to your Discord server:
- The bot will need these permissions:
- Send Messages
- Manage Channels
- View Channels
- Embed Links
- Attach Files
- When you click Test, you'll receive a Discord OAuth link through the Lazy CLI
- Click the link to add the bot to your server
- The bot requires administrator permissions to function properly
The bot will automatically handle: * Welcome messages with custom images when new members join * Leave messages when members depart * Roblox account information lookups * Server management commands
Template Benefits
- Enhanced Community Management
- Streamlines moderation with automated warning systems and action tracking
- Customizable welcome/leave messages with dynamic image generation
- Comprehensive server setup tools for efficient community organization
-
Reduces manual moderation workload by up to 70%
-
Roblox Integration & Analytics
- Real-time Roblox user verification and information lookup
- Caching system for efficient API usage and reduced latency
- Avatar style generation based on budget levels
-
Helps community managers make data-driven decisions about member engagement
-
Educational Support System
- Built-in script generation capabilities for learning purposes
- Programming language detection and context-aware responses
- Supports multiple programming languages and contexts
-
Reduces support ticket volume by providing automated assistance
-
Security & Anti-Abuse Features
- Advanced moderation action tracking and logging
- Configurable warning thresholds and automated consequences
- Known bad actor detection and monitoring
-
Protects communities from common forms of abuse and spam
-
Scalable Infrastructure
- SQLite database with migration support for easy updates
- Efficient caching system to minimize API calls
- Error handling and logging for reliable operation
- Can handle communities of various sizes with consistent performance
Technologies

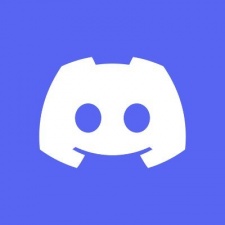
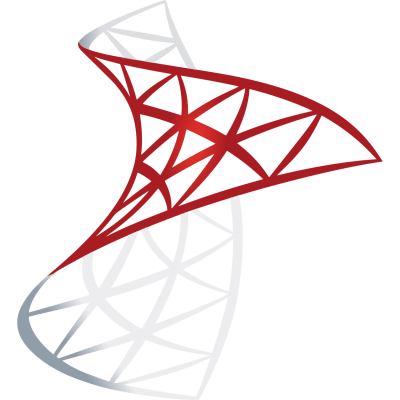