Telegram Web App Bot Template
import os
import logging
from telegram import Update
from telegram.ext import (
Updater,
CommandHandler,
MessageHandler,
CallbackContext,
Filters,
)
TELEGRAM_API_TOKEN = os.getenv('TELEGRAM_API_TOKEN')
logger = logging.getLogger(__name__)
logging.basicConfig(level=logging.INFO, format='%(asctime)s - %(name)s - %(levelname)s - %(message)s')
def start(update: Update, context: CallbackContext) -> None:
message = (
"• ***HOW TO MAKE A TELEGRAM WEB APP WITH LAZY AI*** 😜\n\n"
"**Building your web app**\n"
"-> Create your game as an ordinary website in Lazy AI. Treat it as if you were just creating a game for the browser and not for Telegram at all.\n\n"
"**Building a launcher bot**\n"
"-> Go into the Lazy Builder and say \"Build me a Telegram bot that says hello when people ping it\" and *send*.\n\n"
"-> Get a Telegram bot API key by messaging @BotFather on Telegram and saying /newbot. You will be asked about the name and username of the new bot you want to create, and this process will ultimately leave you with an API key for your new bot.\n\n"
Frequently Asked Questions
What are some potential business applications for this Telegram Web App Bot Template?
The Telegram Web App Bot Template can be used for various business applications, including: - Customer support chatbots that provide instant assistance and guide users to web-based resources - E-commerce bots that showcase products and lead customers to web-based catalogs or stores - Educational platforms that offer interactive lessons or quizzes through a web interface - Event management bots that provide information and link to web-based registration forms - Marketing campaigns that engage users and direct them to promotional web content
How can this template improve user engagement for businesses?
The Telegram Web App Bot Template enhances user engagement by: - Providing a seamless transition from chat interactions to rich web experiences - Offering instant access to web-based content without leaving the Telegram app - Enabling businesses to combine the immediacy of messaging with the functionality of web applications - Allowing for more complex interactions and data input through web forms - Creating a unified brand experience across both chat and web interfaces
What's the advantage of using a Telegram Web App over a traditional website for business?
Using a Telegram Web App with this template offers several advantages: - Increased accessibility as users can access your content directly within Telegram - Higher engagement rates due to the integrated nature of the app within a familiar platform - Simplified user authentication as Telegram handles user identity - Potential for viral growth through Telegram's sharing features - Reduced friction in user acquisition as there's no need to download a separate app
How can I customize the start message in the Telegram Web App Bot Template?
To customize the start message, you can modify the start
function in the main.py
file. Here's an example of how you might change it:
python
def start(update: Update, context: CallbackContext) -> None:
message = (
"Welcome to [Your Business Name]'s Telegram Web App!\n\n"
"Here are some things you can do:\n"
"• Browse our products\n"
"• Check our latest offers\n"
"• Contact customer support\n\n"
"Click the menu button below to get started!"
)
update.message.reply_text(message, parse_mode='Markdown')
This customization allows you to tailor the bot's initial response to your specific business needs and guide users towards your desired actions.
Can I add more command handlers to the Telegram Web App Bot Template?
Yes, you can easily add more command handlers to extend the functionality of your bot. Here's an example of how to add a new command:
```python def help_command(update: Update, context: CallbackContext) -> None: help_text = ( "Here are the available commands:\n" "/start - Begin interaction with the bot\n" "/help - Show this help message\n" "/contact - Get our contact information" ) update.message.reply_text(help_text)
def main(): # ... existing code ... dispatcher.add_handler(CommandHandler("start", start)) dispatcher.add_handler(CommandHandler("help", help_command)) # ... rest of the main function ... ```
By adding these lines, you've created a new /help
command that users can use to get information about available commands. You can continue to add more commands following this pattern to expand your bot's capabilities within the Telegram Web App Bot Template.
Created: | Last Updated:
Introduction to the Telegram Web App Bot Template
This template helps you create a Telegram bot that provides users with steps to build a Telegram web app using Lazy AI. The bot responds with a detailed guide when users interact with it.
Clicking Start with this Template
To get started with the template, click "Start with this Template".
Test
Press the "Test" button to begin the deployment of the app. The Lazy CLI will handle the deployment process.
Using the App
Once the app is deployed, your bot will be online and ready to interact with users. The bot will respond with a detailed guide on how to build a Telegram web app using Lazy AI when users send the `/start` command.
Integrating the App
To link your web app to your bot, follow these steps:
- Open the Telegram app and search for @BotFather or go to https://t.me/botfather.
- Start a chat with BotFather and send the command `/mybots`.
- Select the bot you want to link to your website.
- Click on the "Bot Settings" option.
- Click on "Menu Button".
- Click on "Configure menu button".
- BotFather will ask for a URL - send the URL of your Lazy website.
Code Example
`main.py:
import os
import logging
from telegram import Update
from telegram.ext import (
Updater,
CommandHandler,
MessageHandler,
CallbackContext,
Filters,
)
TELEGRAM_API_TOKEN = os.getenv('TELEGRAM_API_TOKEN')
logger = logging.getLogger(name)
logging.basicConfig(level=logging.INFO, format='%(asctime)s - %(name)s - %(levelname)s - %(message)s')
def start(update: Update, context: CallbackContext) -> None:
message = (
"• HOW TO MAKE A TELEGRAM WEB APP WITH LAZY AI 😜\n\n"
"Building your web app\n"
"-> Create your game as an ordinary website in Lazy AI. Treat it as if you were just creating a game for the browser and not for Telegram at all.\n\n"
"Building a launcher bot\n"
"-> Go into the Lazy Builder and say \"Build me a Telegram bot that says hello when people ping it\" and send.\n\n"
"-> Get a Telegram bot API key by messaging @BotFather on Telegram and saying /newbot. You will be asked about the name and username of the new bot you want to create, and this process will ultimately leave you with an API key for your new bot.\n\n"
"-> Copy the API key @BotFather gave you into the Env Secrets tab in the Lazy Builder (there will be an Env Secret ready for you to fill)\n\n"
"-> Run your Lazy app, and your bot will be online\n\n"
"Linking your web app to your bot\n"
"-> Go back into Telegram and say /mybots (to BotFather)\n\n"
"-> Select the bot you want to link to your website\n\n"
"-> Click on the \"Bot Settings\" option\n\n"
"-> Click on \"Menu Button\"\n\n"
"-> Click on \"Configure menu button\"\n\n"
"-> Botfather will ask for you to send it a URL - send the URL of your Lazy website (domain doesn't matter as the bot will not display the domain anyway)\n\n"
"Video tutorial coming soon!!!"
)
update.message.reply_text(message, parse_mode='Markdown')
def error_handler(update: Update, context: CallbackContext) -> None:
logger.error(f"Update {update} caused error {context.error}")
update.message.reply_text("An error occurred. Please try again later.")
def main():
if not TELEGRAM_API_TOKEN:
print("🚨 Oops! The TELEGRAM_API_TOKEN 🔑 environment variable is missing. 🚨")
print("Let's fix that together! 😊 Here's how:\n" +
"1. 🌐 Open the Telegram app and search for the BotFather or go to https://t.me/botfather 🌐\n" +
"2. ✏️ Start a chat with BotFather and send the command /newbot. ✏️\n" +
"3. 🤖 Follow the instructions to set up your new bot, providing a name and username. 🤖\n" +
"4. 🔑 Once your bot is created, BotFather will provide you with a TELEGRAM_API_TOKEN. 🔑\n" +
"5. 📋 Copy this token. 📋\n" +
"6. 🔐 Set this value in the Env Secrets tab 🔐\n" +
"For more information, refer to the Telegram Bot API documentation: https://core.telegram.org/bots#3-how-do-i-create-a-bot. 🚀")
return
updater = Updater(TELEGRAM_API_TOKEN)
dispatcher = updater.dispatcher
dispatcher.add_handler(CommandHandler("start", start))
dispatcher.add_error_handler(error_handler)
logger.info("Bot has successfully connected and started polling. 🚀")
updater.start_polling()
updater.idle()
if name == "main":
main()
requirements.txt:
python-telegram-bot==13.13`
For more information, refer to the Telegram Bot API documentation: https://core.telegram.org/bots#3-how-do-i-create-a-bot.
Template Benefits
-
Rapid Prototyping: This template enables businesses to quickly create and deploy a Telegram bot that can serve as a launcher for web applications, significantly reducing development time and costs.
-
Customer Engagement: By providing an interactive guide on how to build Telegram web apps, this bot can engage users and potentially convert them into active developers or customers for the Lazy AI platform.
-
Educational Tool: The bot serves as an educational resource, teaching users how to create Telegram web apps. This can be valuable for businesses offering coding courses or development services.
-
Lead Generation: As users interact with the bot to learn about building web apps, businesses can collect valuable data on potential customers interested in app development, creating opportunities for targeted marketing.
-
Scalable Platform Introduction: The template provides a scalable way to introduce users to the Lazy AI platform, potentially increasing user adoption and expanding the customer base for businesses offering AI-powered development tools.
Technologies
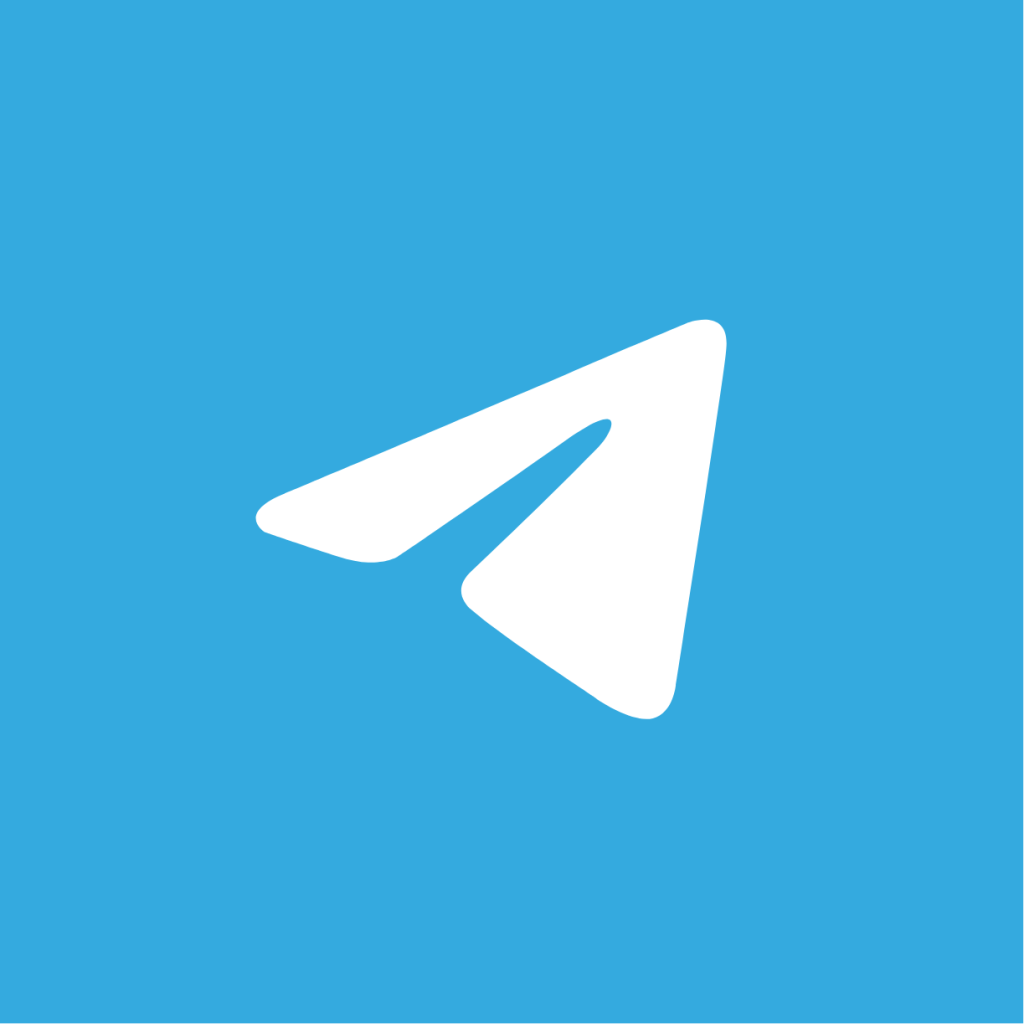