OANDA Trading Bot CLI
import logging
import os
import time
import pandas as pd
import numpy as np
from oandapyV20 import API
import oandapyV20.endpoints.orders as orders
import oandapyV20.endpoints.trades as trades
import oandapyV20.endpoints.pricing as pricing
from oandapyV20.endpoints.accounts import AccountSummary, AccountList
from oandapyV20.exceptions import V20Error
from decimal import Decimal, ROUND_HALF_UP
import json
import threading
from datetime import datetime, timedelta
logger = logging.getLogger(__name__)
logging.basicConfig(level=logging.INFO)
class ImprovedOandaTradingBot:
def __init__(self, instruments=None, initial_balance=100000):
if instruments is None:
instruments = ["EUR_JPY"]
# Fixed take profit and stop loss in dollars
Frequently Asked Questions
What are the main benefits of using the OANDA Trading Bot CLI for forex trading?
The OANDA Trading Bot CLI offers several advantages for forex traders: - Automated trading execution based on predefined strategies - Real-time monitoring of open positions and account balance - Dynamic calculation of take profit and stop loss levels using ATR - Risk management through favorable risk-reward ratio checks - Separate threads for price updates, P&L monitoring, and order management, ensuring efficient operation
This CLI tool allows traders to implement and test strategies without constant manual intervention, potentially improving trading consistency and reducing emotional decision-making.
Can the OANDA Trading Bot CLI be customized for different trading strategies?
Yes, the OANDA Trading Bot CLI is designed to be customizable. Traders can modify the calculate_indicators
method to add or change technical indicators, and adjust the trading logic in the main
function. For example, you could implement a moving average crossover strategy by adding the following code to the calculate_indicators
method:
```python def calculate_indicators(self, data): # Existing code...
# Add moving average crossover
data['SMA_short'] = data['Close'].rolling(window=10).mean()
data['SMA_long'] = data['Close'].rolling(window=50).mean()
return data
```
Then, update the trading logic in main
:
python
if last_row['SMA_short'] > last_row['SMA_long']:
bot.execute_trade('buy', data)
elif last_row['SMA_short'] < last_row['SMA_long']:
bot.execute_trade('sell', data)
This flexibility allows traders to implement various strategies using the OANDA Trading Bot CLI as a foundation.
How does the OANDA Trading Bot CLI manage risk in trading operations?
The OANDA Trading Bot CLI incorporates several risk management features: - Dynamic take profit and stop loss levels based on the Average True Range (ATR) - Risk-reward ratio calculation to ensure favorable trade setups (minimum 2:1 ratio) - Spread checks to avoid trading when the spread is too wide relative to the take profit target - Position size limits (maximum of 2 open buy or sell orders) - Continuous monitoring of open positions with automatic closure at take profit or stop loss levels
These features help manage risk by ensuring that trades are only executed under favorable conditions and that losses are limited while allowing profits to run.
How can I integrate the OANDA Trading Bot CLI with external data sources?
The OANDA Trading Bot CLI can be extended to work with external data sources. You can modify the main
function to fetch data from APIs or other sources instead of using mock data. Here's an example of how you might integrate with an external API:
```python import requests
def fetch_external_data(symbol, timeframe): url = f"https://api.example.com/data?symbol={symbol}&timeframe={timeframe}" response = requests.get(url) if response.status_code == 200: return pd.DataFrame(response.json()) else: raise Exception("Failed to fetch data from external API")
def main(): bot = ImprovedOandaTradingBot()
# Fetch data from external API
data = fetch_external_data("EUR_USD", "1H")
# Calculate indicators and execute trading strategy
data = bot.calculate_indicators(data)
# Rest of the main function...
```
This approach allows the OANDA Trading Bot CLI to use real-time or historical data from various sources, enhancing its versatility and potential applications.
What are some potential applications of the OANDA Trading Bot CLI beyond individual trading?
The OANDA Trading Bot CLI has several potential applications beyond individual trading: - Algorithmic Trading Firms: Can use it as a base for developing and testing more complex trading algorithms - Educational Institutions: Can utilize it to teach students about algorithmic trading and financial market dynamics - Financial Research: Researchers can modify the bot to backtest trading strategies on historical data - Portfolio Management: Asset managers can adapt the bot to automate parts of their forex portfolio management process - Fintech Startups: Can use the bot as a starting point for developing more comprehensive trading platforms
The modular nature of the OANDA Trading Bot CLI makes it a versatile tool that can be adapted for various financial industry applications, from education to professional trading operations.
Created: | Last Updated:
Here's a step-by-step guide for using the OANDA Trading Bot CLI template:
Introduction
This template provides a CLI-based OANDA Trading Bot for monitoring and managing trading orders. It includes separated API calls for open P&L, take profit, and stop loss. The bot uses the OANDA API to execute trades, monitor positions, and manage orders for specified instruments.
Getting Started
- Click "Start with this Template" to begin using the OANDA Trading Bot CLI template in the Lazy Builder interface.
Initial Setup
Before running the bot, you need to set up your OANDA API token as an environment secret:
- Go to the Environment Secrets tab in the Lazy Builder.
- Add a new secret with the key
OANDA_API_TOKEN
. - To get your OANDA API token:
- Log in to your OANDA account
- Navigate to the "Manage API Access" section
- Generate a new API token or copy your existing one
- Paste your OANDA API token as the value for the
OANDA_API_TOKEN
secret.
Test the App
- Click the "Test" button in the Lazy Builder interface to deploy and run the OANDA Trading Bot.
Using the App
Once the app is running, it will automatically:
- Initialize the OANDA account
- Start separate threads for price fetching, P&L monitoring, and order management
- Execute trades based on the implemented strategy
- Monitor and update open positions
- Log important information and errors
The bot will continue running and managing trades until you stop it. You can monitor its activity through the logs provided in the Lazy CLI.
Customizing the Bot
To customize the bot's behavior, you can modify the following parameters in the ImprovedOandaTradingBot
class:
instruments
: List of trading instruments (default is["EUR_JPY"]
)initial_balance
: Initial account balance (default is 100000)take_profit
: Fixed take profit amount in dollars (default is $1)stop_loss
: Fixed stop loss amount in dollars (default is $20)position_size
: Size of each trading position (default is 2.5)atr_period
: Period for ATR calculation (default is 14)atr_multiplier_tp
: ATR multiplier for take profit (default is 2.0)atr_multiplier_sl
: ATR multiplier for stop loss (default is 1.0)
To change these parameters, modify the values in the __init__
method of the ImprovedOandaTradingBot
class.
Important Notes
- The bot uses the OANDA practice environment by default. To switch to a live account, change the
environment
parameter in theAPI
initialization to "live". - The bot currently supports trading EUR_JPY and XAU_USD instruments. To add more instruments, update the
validate_instruments
method. - The trading strategy is based on Stochastic Oscillator, RSI, and Volume indicators. You can modify the
execute_trade
method to implement your own trading logic. - Always monitor the bot's performance and adjust parameters as needed to manage risk effectively.
Remember that trading involves financial risk. Use this bot responsibly and consider testing thoroughly with a practice account before using it with real funds.
Here are 5 key business benefits for this OANDA Trading Bot CLI template:
Template Benefits
-
Automated Trading Strategy: The template implements an automated trading strategy using technical indicators like ATR, Stochastic Oscillator, and RSI. This allows businesses to execute trades 24/7 without constant manual monitoring, potentially increasing trading opportunities and profits.
-
Risk Management: The bot incorporates dynamic take profit and stop loss levels based on market volatility (ATR), as well as a risk-reward ratio check. This helps protect capital and manage downside risk, which is crucial for long-term trading success.
-
Real-time Monitoring: With separate threads for price fetching, P&L monitoring, and order management, the bot provides real-time updates on market conditions and trade performance. This allows for quick decision-making and strategy adjustments.
-
Scalability: The template is designed to handle multiple trading instruments simultaneously. This scalability allows businesses to diversify their trading portfolio and potentially increase overall returns.
-
Performance Tracking: The bot includes functionality to monitor account balance, total P&L, and individual trade performance. This detailed tracking enables businesses to analyze trading strategy effectiveness and make data-driven improvements over time.
Technologies
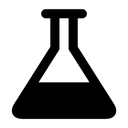
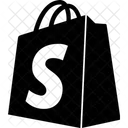

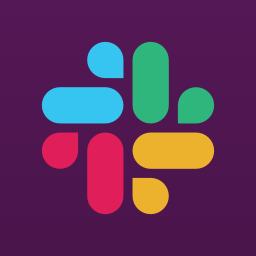
