NYx
import os
import discord
from discord import app_commands
from abilities import apply_sqlite_migrations
from models import Base, engine
def generate_oauth_link(client_id):
base_url = "https://discord.com/api/oauth2/authorize"
redirect_uri = "http://localhost"
scope = "bot"
permissions = "8" # Administrator permission for simplicity, adjust as needed.
return f"{base_url}?client_id={client_id}&permissions={permissions}&scope={scope}"
intents = discord.Intents.default()
intents.messages = True
intents.message_content = True
intents.members = True # Enable member intents for welcome messages
bot = discord.Client(intents=intents, activity=discord.CustomActivity("Welcoming new members!"))
tree = app_commands.CommandTree(bot)
@bot.event
async def on_ready():
Frequently Asked Questions
How can the Welcomer Bot for Discord benefit my online community?
The Welcomer Bot for Discord can significantly enhance your community's onboarding experience. By automatically greeting new members with a personalized welcome message, it creates a warm and inviting atmosphere from the moment they join. This initial positive interaction can increase member engagement and retention, making newcomers feel valued and more likely to participate in your community.
Can I customize the welcome message for different types of communities?
Absolutely! The Welcomer Bot for Discord is designed to be flexible. While the current template uses a basic welcome message, you can easily modify it to suit your community's tone and needs. For example, you could add specific instructions, community rules, or even incorporate emojis and GIFs to make the welcome more engaging. This customization allows you to maintain your community's unique culture from the first interaction.
How does the Welcomer Bot for Discord compare to paid community management tools?
The Welcomer Bot for Discord offers a cost-effective solution for automating member greetings, which is a key feature of many paid community management tools. While it may not have all the advanced features of premium services, it provides a solid foundation that can be expanded upon. It's an excellent starting point for communities looking to enhance their member experience without a significant financial investment.
How can I modify the Welcomer Bot to send welcome messages to a specific channel instead of the system channel?
You can easily modify the Welcomer Bot to send messages to a specific channel. In the on_member_join
function, replace the welcome_channel = member.guild.system_channel
line with code to get your desired channel. For example:
python
@bot.event
async def on_member_join(member):
welcome_channel = discord.utils.get(member.guild.channels, name="welcome")
if welcome_channel:
welcome_message = f"Welcome to the server, {member.mention}! 👋"
await welcome_channel.send(welcome_message)
This code will send the welcome message to a channel named "welcome". Make sure to create this channel in your Discord server.
Can the Welcomer Bot be extended to include other features like role assignment?
Yes, the Welcomer Bot for Discord can be extended to include additional features like role assignment. You can modify the on_member_join
event to assign roles. Here's an example of how you might do this:
```python @bot.event async def on_member_join(member): welcome_channel = member.guild.system_channel if welcome_channel: welcome_message = f"Welcome to the server, {member.mention}! 👋" await welcome_channel.send(welcome_message)
# Assign a role
role = discord.utils.get(member.guild.roles, name="New Member")
if role:
await member.add_roles(role)
print(f"Assigned the 'New Member' role to {member.name}")
```
This code assigns a "New Member" role to new joiners, in addition to sending the welcome message. Remember to create the "New Member" role in your Discord server for this to work.
Created: | Last Updated:
Here's a step-by-step guide on how to use the Welcomer Bot for Discord template:
Introduction
The Welcomer Bot for Discord is a simple bot that automatically sends a welcome message to new members in a designated channel. This template provides a basic structure for creating a Discord bot with welcome functionality.
Getting Started
- Click "Start with this Template" to begin using the Welcomer Bot template in the Lazy Builder interface.
Initial Setup
Before testing the bot, you need to set up some environment secrets:
- Go to the Discord Developer Portal (https://discord.com/developers/applications).
- Create a new application and navigate to the 'Bot' section.
- Click 'Add Bot' and confirm by clicking 'Yes, do it!'.
- Under the 'TOKEN' section, click 'Copy' to get your BOT_TOKEN.
- Navigate to the 'OAuth2' section, under 'CLIENT ID', click 'Copy' to get your CLIENT_ID.
- In the Lazy Builder, go to the Environment Secrets tab.
- Add two new secrets:
- Key:
CLIENT_ID
, Value: [Your copied Client ID] - Key:
BOT_TOKEN
, Value: [Your copied Bot Token] - In the Discord Developer Portal, make sure to enable the 'Server Members Intent' in your bot settings. This is required for welcome messages.
Testing the Bot
- Click the "Test" button in the Lazy Builder interface to start the deployment process.
- The Lazy CLI will display a message with an OAuth link to invite your bot to a Discord server.
- Copy this link and open it in a web browser.
- Select the server you want to add the bot to and grant the necessary permissions.
Using the Bot
Once the bot is added to your server:
- The bot will automatically send a welcome message in the server's system channel when a new member joins.
- You can use the
/echo
command in any channel where the bot is present. For example, typing/echo Hello, world!
will make the bot respond with "Hello, world!".
Customizing the Bot
To customize the bot's functionality:
- Modify the welcome message in the
on_member_join
event handler in themain.py
file. - Add new commands by creating additional functions decorated with
@tree.command()
. - Implement more complex features using the discord.py library and SQLAlchemy for database operations if needed.
Remember that any changes you make will require redeploying the bot using the "Test" button in the Lazy Builder interface.
Here are 5 key business benefits for this Discord Welcomer Bot template:
Template Benefits
-
Enhanced User Onboarding: Automatically greets new members, making them feel welcome and improving their initial experience with the community or brand.
-
Increased Engagement: By providing a personalized welcome message, the bot encourages new members to interact with the server, potentially leading to higher retention rates.
-
Time-Saving Automation: Eliminates the need for manual welcoming, allowing community managers to focus on more strategic tasks and interactions.
-
Scalable Community Management: Efficiently handles welcoming duties even as the server grows, maintaining a consistent onboarding experience for all new members.
-
Customizable Brand Presence: The welcome message can be tailored to reflect the brand's voice and values, reinforcing brand identity from the moment a new member joins.
Technologies
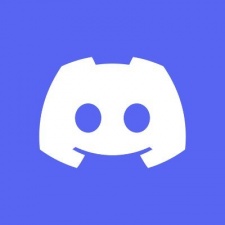
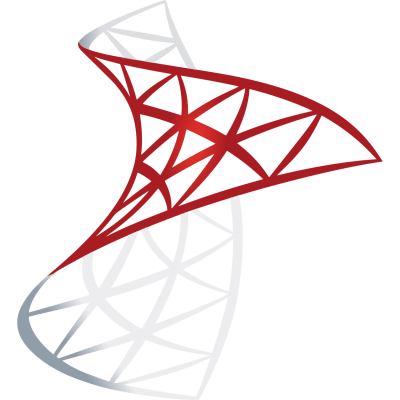