by Muhammad
Notes App With Database
from models import Note
from flask import url_for
from flask import redirect
from flask import request
from flask import jsonify # Added import for jsonify
import logging
from flask import Flask, render_template
from gunicorn.app.base import BaseApplication
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
app = Flask(__name__)
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///users.db'
app.config['SECRET_KEY'] = 'replace-with-a-secret-key'
from models import db, User
db.init_app(app)
with app.app_context():
db.create_all()
Frequently Asked Questions
How can the Notes App With Database benefit small businesses?
The Notes App With Database can greatly benefit small businesses by providing a centralized platform for employees to create, store, and manage important information. Teams can use it for project planning, meeting notes, task lists, and idea brainstorming. The app's user authentication system ensures that each employee's notes remain private and secure, while still allowing for easy access from any device with internet connectivity.
Can the Notes App With Database be customized for specific industry needs?
Absolutely! The Notes App With Database is built with Flask, which makes it highly customizable. For example, a law firm could add categories for different case types, while a marketing agency could include fields for campaign ideas and client information. The app's modular structure allows developers to easily extend its functionality to meet specific industry requirements without compromising its core note-taking capabilities.
How does the Notes App With Database compare to cloud-based note-taking solutions in terms of data privacy?
The Notes App With Database offers enhanced data privacy compared to many cloud-based solutions. Since it can be hosted on a company's own servers, businesses have complete control over their data. This is particularly important for industries dealing with sensitive information, such as healthcare or finance. Additionally, the app uses secure password hashing and user authentication, ensuring that each user's notes are protected from unauthorized access.
How can I add rich text formatting to notes in the Notes App With Database?
To add rich text formatting, you can integrate a WYSIWYG (What You See Is What You Get) editor like TinyMCE or CKEditor into the app. Here's an example of how you might integrate TinyMCE into the create_note.html
template:
```html
```
This integration would allow users to format their notes with bold, italic, lists, and even insert images directly in the Notes App With Database.
How can I implement a search functionality in the Notes App With Database?
To add a search functionality, you can modify the note_dashboard
route in main.py
to accept a search query and filter the notes accordingly. Here's an example implementation:
```python from flask import request
@app.route("/note_dashboard", methods=["GET"]) def note_dashboard(): user_id = session.get('user_id') if not user_id: return redirect(url_for('signin')) user = User.query.get(user_id) if user: search_query = request.args.get('search', '') if search_query: notes = Note.query.filter_by(user_id=user.id).filter( (Note.title.ilike(f'%{search_query}%')) | (Note.content.ilike(f'%{search_query}%')) ).all() else: notes = Note.query.filter_by(user_id=user.id).all() return render_template("note_dashboard.html", username=user.username, notes=notes, search_query=search_query) else: return "User not found", 404 ```
Then, add a search form to the note_dashboard.html
template:
```html
```
This implementation allows users to search their notes in the Notes App With Database by title or content, enhancing the app's usability for those with many notes.
Created: | Last Updated:
Introduction to the Notes App With Database Template
The Notes App With Database template is a powerful tool for builders looking to create an application that allows users to manage their notes. This app provides functionality for users to create, edit, delete, and view their notes, all stored within a database. With this template, you can set up a full-fledged notes application without worrying about the complexities of backend development or deployment.
Getting Started
To begin using this template, simply click on "Start with this Template" on the Lazy platform. This will pre-populate the code in the Lazy Builder interface, so you won't need to copy or paste any code manually.
Initial Setup
Before testing the application, you'll need to set up a secret key for your application. This key is used to keep your sessions secure. Here's how to set it up:
- Go to the Environment Secrets tab within the Lazy Builder.
- Create a new secret with the key
SECRET_KEY
. - Enter a secure and unique value for this key. It can be any string, but it should be difficult to guess.
Test: Pressing the Test Button
Once you've set up your secret key, you can test the application by clicking the "Test" button. This will deploy your app and launch the Lazy CLI. If the code requires any user input, you will be prompted to provide it through the Lazy CLI.
Entering Input
If the application requires user input, such as a username or password for signing up or signing in, you will be prompted to enter this information after pressing the "Test" button. Follow the prompts in the CLI to input the necessary information.
Using the App
After deployment, the Lazy platform will provide you with a dedicated server link to access your notes application. Here's how to navigate the interface:
- Visit the provided server link to view the home page of the Notes App.
- Sign up for an account or sign in if you already have one.
- Once signed in, you can create a new note by clicking on "Create Note".
- Fill in the title and content for your note and click "Save".
- Your note will now be visible on the dashboard, where you can edit or delete it as needed.
- To log out, simply click the "Log Out" button.
Integrating the App
If you wish to integrate this notes application into another service or frontend, you can use the provided server link as the API endpoint. For example, you can make HTTP requests to create, retrieve, update, or delete notes. Here's a sample request to create a new note:
`POST /create_note HTTP/1.1
Host: [Your Server Link]
Content-Type: application/x-www-form-urlencoded
title=Sample Note&content=This is a sample note content.`
And here's a sample response you might receive after creating a new note:
`HTTP/1.1 200 OK
Content-Type: application/json
{
"success": true,
"message": "Note created successfully."
}`
Remember to replace "[Your Server Link]" with the actual link provided by the Lazy platform after deployment.
By following these steps, you can easily set up and use the Notes App With Database template to create a personalized note-taking application for your users.
Here are 5 key business benefits for this Notes App template:
Template Benefits
-
Improved Productivity: Allows employees to quickly capture, organize and access important information, ideas and to-do items, enhancing personal and team productivity.
-
Secure Information Management: Provides a centralized and secure platform for storing sensitive business notes and information, with user authentication and individual accounts.
-
Collaboration Enhancement: Enables easy sharing and collaboration on notes between team members, facilitating better communication and knowledge sharing within an organization.
-
Customizable for Business Needs: The template can be easily adapted to specific business requirements, such as adding categories, tags, or integrating with other business systems.
-
Cost-Effective Solution: Offers a ready-to-use notes application that can be quickly deployed, saving development time and costs compared to building a custom solution from scratch.
Technologies
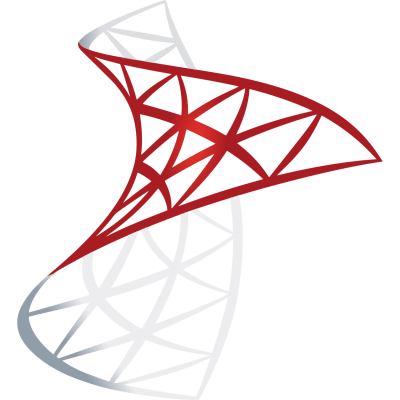