Image Organizer
import logging
from gunicorn.app.base import BaseApplication
from app_init import create_initialized_flask_app
# Flask app creation should be done by create_initialized_flask_app to avoid circular dependency problems.
app = create_initialized_flask_app()
# Setup logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
class StandaloneApplication(BaseApplication):
def __init__(self, app, options=None):
self.application = app
self.options = options or {}
super().__init__()
def load_config(self):
# Apply configuration to Gunicorn
for key, value in self.options.items():
if key in self.cfg.settings and value is not None:
self.cfg.set(key.lower(), value)
def load(self):
Frequently Asked Questions
How can the Image Organizer application benefit businesses in the creative industry?
The Image Organizer application can significantly benefit businesses in the creative industry by streamlining their image management processes. It allows for quick scanning and organization of large image libraries, which is crucial for design agencies, marketing firms, and photography studios. The advanced search capabilities and AI-based classification (once fully implemented) will enable creative professionals to find the exact images they need quickly, saving time and improving productivity. Additionally, the ability to generate datasets can be valuable for businesses looking to develop AI-powered tools or conduct visual trend analysis.
Can the Image Organizer be customized for specific industry needs?
Yes, the Image Organizer is designed with flexibility in mind. While the current template focuses on general image organization and classification of feet and cosplay images, it can be easily adapted to suit specific industry needs. For example, a fashion retailer could customize the application to categorize images by clothing type, color, or style. A real estate agency could modify it to organize property images by features or location. The modular structure of the Image Organizer allows for easy integration of new classification models and filters tailored to particular business requirements.
How does the Image Organizer contribute to data privacy and security for businesses?
The Image Organizer prioritizes data privacy and security by operating locally on the user's machine. This means that sensitive images never leave the company's network, which is crucial for businesses dealing with confidential visual data. The application doesn't require internet connectivity for its core functionalities, further enhancing security. Additionally, the use of SQLite as the database (as seen in the app_init.py
file) ensures that all metadata is stored locally. For businesses requiring additional security measures, the Image Organizer's codebase can be extended to include encryption and access control features.
How can I add a new image filter to the Image Organizer application?
To add a new image filter to the Image Organizer, you'll need to modify the home.js
file. Here's an example of how to add a filter for high-resolution images:
``javascript
function createFilters() {
filterContainer.innerHTML =
// ... existing filters ...
`;
filterContainer.addEventListener('click', (event) => {
if (event.target.classList.contains('filter-btn')) {
const filter = event.target.dataset.filter;
let filteredImages;
switch (filter) {
// ... existing cases ...
case 'high-res':
filteredImages = allImages.filter(img => img.size > 1024 * 1024); // Filter images larger than 1MB
break;
}
displayResults(filteredImages);
}
});
} ```
You'll also need to ensure that the imageInfo
object in the file scanning loop includes the necessary data for your new filter.
How can I extend the Image Organizer to support additional image formats?
The Image Organizer currently supports common image formats like JPEG, PNG, GIF, BMP, and WebP. To add support for additional formats, you need to modify the file type checking in the home.js
file. Here's an example of how to add support for TIFF images:
```javascript folderInput.addEventListener('change', async (event) => { const files = Array.from(event.target.files).filter(file => ['image/jpeg', 'image/png', 'image/gif', 'image/bmp', 'image/webp', 'image/tiff'].includes(file.type) );
// ... rest of the function ...
}); ```
Additionally, you may need to update the server-side code to handle the new format if you implement any server-side processing. Keep in mind that browser support for different image formats may vary, so you might need to use appropriate libraries or fallbacks for full compatibility across all platforms.
Created: | Last Updated:
Here's a step-by-step guide for using the Image Organizer App template:
Introduction
The Image Organizer App is a desktop application designed to scan, classify, and organize local images. It offers advanced search capabilities and can generate datasets for AI projects. This template provides a foundation for building an image organization tool with a user-friendly web interface.
Getting Started
To begin using this template:
- Click the "Start with this Template" button in the Lazy Builder interface.
Test the Application
Once you've started with the template:
- Click the "Test" button in the Lazy Builder interface.
- This will initiate the deployment process and launch the Lazy CLI.
Using the App
After the deployment is complete, you'll be provided with a server link to access the web interface. Here's how to use the application:
- Open the provided link in your web browser.
- You'll see the main interface with the title "Local Image Scanner and Organizer".
- Click the "Choose Folder" button to select a folder containing images you want to scan and organize.
- The app will process the images in the selected folder, displaying a progress bar during the scan.
- Once the scan is complete, you'll see a list of processed images and a summary.
- Use the filter buttons to view specific categories of images:
- All Images
- Feet Images
- Non-Feet Images
- Cosplay Images
- Cosplay Feet Images
- The filtered results will update in real-time as you select different categories.
- To download the filtered images, click the "Download Filtered Images" button (Note: This functionality is not yet implemented in the current version).
Limitations and Future Improvements
Please note the following limitations of the current template:
- The AI-based image classification is currently simulated with random values. In a real implementation, this would be replaced with actual AI models for accurate classification.
- The download functionality for filtered images is not yet implemented.
- The app currently only scans and organizes images within a single selected folder. Recursive folder scanning is not supported in this version.
Integrating the App
This web-based application runs standalone and doesn't require integration with external services. However, if you want to extend its functionality or integrate it with other systems, you can modify the Flask backend (main.py
, routes.py
, and app_init.py
) and the frontend JavaScript (home.js
) as needed.
By following these steps, you'll have a basic image organization app up and running. You can further customize and expand its features based on your specific requirements.
Template Benefits
-
Efficient Image Management: This template provides a robust foundation for building an image organization tool, allowing businesses to efficiently scan, categorize, and manage large volumes of digital images. This can significantly improve productivity for companies dealing with extensive image libraries.
-
AI-Ready Dataset Creation: The template includes functionality for filtering and categorizing images, which can be leveraged to create custom datasets for AI training. This is particularly valuable for businesses looking to develop machine learning models for image recognition or classification tasks.
-
User-Friendly Interface: With a responsive design that works on both desktop and mobile devices, this template offers a user-friendly interface for image management. This can improve user adoption rates and reduce the learning curve for employees using the tool.
-
Scalable Architecture: The use of Flask, SQLAlchemy, and Gunicorn provides a scalable backend architecture. This allows businesses to start small and easily scale up as their image management needs grow, without requiring a complete overhaul of the system.
-
Customizable Filtering: The template includes a flexible filtering system that can be easily customized to meet specific business needs. This allows companies to tailor the image organization process to their unique requirements, improving overall efficiency and relevance of the tool for various industries or use cases.
Technologies
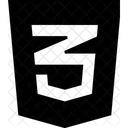
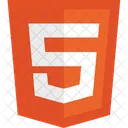