by Lazy Sloth
FALLBACK | Flask, HTML, JS and Tailwind Based Website
import logging
from gunicorn.app.base import BaseApplication
from app_init import create_app
app = create_app()
# Setup logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
class StandaloneApplication(BaseApplication):
def __init__(self, app, options=None):
self.application = app
self.options = options or {}
super().__init__()
def load_config(self):
# Apply configuration to Gunicorn
for key, value in self.options.items():
if key in self.cfg.settings and value is not None:
self.cfg.set(key.lower(), value)
def load(self):
return self.application
Frequently Asked Questions
What types of businesses would benefit most from using the FALLBACK template?
The FALLBACK template is versatile and can benefit a wide range of businesses. It's particularly well-suited for startups, small to medium-sized enterprises, and freelancers who need a professional-looking website quickly. Its clean design and responsive layout make it ideal for service-based businesses, portfolios, landing pages, and simple e-commerce sites. The template's use of Tailwind CSS and Flowbite components allows for easy customization to match various brand identities.
How can the FALLBACK template improve a company's online presence?
The FALLBACK template can significantly enhance a company's online presence in several ways: - It provides a modern, responsive design that works well on all devices, improving user experience. - The clean layout and customizable components allow for clear presentation of company information and services. - The template's performance optimization helps with faster load times, which can improve search engine rankings. - Its modular structure makes it easy to add new pages or sections as the business grows, ensuring the website can evolve with the company.
What are the cost implications of using the FALLBACK template compared to custom web development?
Using the FALLBACK template can lead to significant cost savings compared to custom web development. It provides a solid foundation that reduces development time and effort. While custom development can cost thousands of dollars and take weeks or months, the FALLBACK template allows businesses to have a professional website up and running in a fraction of the time and cost. However, some customization may still be needed to fully align with specific business needs, but this would still be more cost-effective than starting from scratch.
How can I add a new page to the FALLBACK template?
To add a new page to the FALLBACK template, you'll need to create a new HTML file and add a corresponding route in the routes.py
file. Here's an example:
How can I customize the header menu in the FALLBACK template?
To customize the header menu in the FALLBACK template, you'll need to modify both the _desktop_header.html
and _mobile_header.html
files. Here's an example of how to add a new "About" link:
In _desktop_header.html
:
```html
```
In _mobile_header.html
:
```html
```
Remember to create the corresponding route and template for the new page as explained in the previous question.
Created: | Last Updated:
Introduction to the Template
This template provides a starting point for a styled website using Flask, HTML, JavaScript, and Tailwind CSS. It includes a responsive header that adapts to both mobile and desktop views, and it is pre-configured with Tailwind and Flowbite for easy styling. This template is ideal for building visually appealing web pages quickly.
Clicking Start with this Template
To get started with this template, click the "Start with this Template" button.
Test
Press the "Test" button to deploy the app and launch the Lazy CLI. This will start the application and make it accessible for further configuration and testing.
Using the App
Once the app is deployed, you can access the main page of your website. The header will adapt based on the screen size: - On mobile devices, a menu button will appear. Clicking this button will toggle the visibility of the mobile menu. - On desktop devices, a navigation bar will be displayed.
Mobile Header
<div class="mobile-menu-button">
<button id="mobile-menu-button" class="inline-flex items-center p-2 text-sm text-gray-500 rounded-lg hover:bg-gray-100 focus:outline-none focus:ring-2 focus:ring-gray-200" aria-controls="mobile-menu" aria-expanded="false">
<span class="sr-only">Open main menu</span>
<svg class="w-6 h-6" fill="none" stroke="currentColor" viewBox="0 0 24 24" xmlns="http://www.w3.org/2000/svg">
<path stroke-linecap="round" stroke-linejoin="round" stroke-width="2" d="M4 6h16M4 12h16m-7 6h7"></path>
</svg>
</button>
</div>
<div id="mobile-menu" class="hidden w-full absolute top-16 left-0 bg-white shadow-md">
<ul class="flex flex-col space-y-2 p-4">
<li><a href="/" class="block py-2 pr-4 pl-3 text-gray-700 border-b border-gray-100 hover:bg-gray-50">Home</a></li>
</ul>
</div>
Desktop Header
<nav class="desktop-nav">
<ul class="flex space-x-4">
<li><a href="/" class="font-mono text-gray-900 py-2 px-4 rounded-md bg-blue-500 hover:bg-blue-600 text-white transition duration-300">Home</a></li>
</ul>
</nav>
Header
<header class="text-gray-600 body-font shadow-lg">
<div class="container mx-auto flex flex-wrap p-5 flex-row items-center justify-between">
<div class="flex items-center">
<img src="https://storage.googleapis.com/lazy-icons/not-found-placeholder.webp" class="rounded-full w-10 h-10 mr-2">
<span class="flex title-font font-medium items-center text-gray-900 text-xl">App Name</span>
</div>
<div class="hidden md:block">
{% include 'partials/_desktop_header.html' %}
</div>
<div class="md:hidden">
{% include 'partials/_mobile_header.html' %}
</div>
</div>
</header>
Integrating the App
To integrate this app into your project, follow these steps:
1. **Include the Header in Your HTML Files**:
Ensure that the header is included in your HTML files where you want the navigation to appear. For example, in `home.html`:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<base href="/">
<link rel="stylesheet" href="/static/css/styles.css" />
<link rel="icon" href="https://storage.googleapis.com/lazy-icons/not-found-placeholder.webp" type="image/x-icon">
<title>App Name</title>
</head>
<body class="bg-gray-100 font-sans leading-normal tracking-normal">
{% include 'partials/_header.html' %}
<div class="container mx-auto px-4 mt-8 min-h-screen">
<div class="text-center py-10">
<!-- Content Goes Here -->
</div>
</div>
<script src="/static/js/script.js"></script>
</body>
</html>
2. **JavaScript for Mobile Menu**:
Ensure the JavaScript for toggling the mobile menu is included. This is already provided in `script.js`:
`document.addEventListener('DOMContentLoaded', () => {
const mobileMenuButton = document.getElementById('mobile-menu-button');
const mobileMenu = document.getElementById('mobile-menu');
mobileMenuButton.addEventListener('click', () => {
mobileMenu.classList.toggle('hidden');
});
// Close mobile menu when clicking outside
document.addEventListener('click', (event) => {
if (!mobileMenu.contains(event.target) && !mobileMenuButton.contains(event.target)) {
mobileMenu.classList.add('hidden');
}
});
});`
3. **Styling with Tailwind CSS**:
The template uses Tailwind CSS for styling. Ensure that the Tailwind CSS and Flowbite CSS are included in your `styles.css`:
`@import url("https://cdn.jsdelivr.net/npm/tailwindcss@3.4.3/base.min.css");
@import url("https://cdnjs.cloudflare.com/ajax/libs/flowbite/2.3.0/flowbite.min.css");
/ Custom styles /
/ No custom styles needed, using Tailwind classes /`
By following these steps, you will have a fully functional and styled website header that adapts to both mobile and desktop views.
Template Benefits
-
Rapid Prototyping: This template provides a ready-to-use structure for quickly building and deploying web applications, allowing businesses to prototype ideas and launch MVPs (Minimum Viable Products) faster.
-
Responsive Design: With built-in mobile and desktop layouts, the template ensures a consistent user experience across devices, potentially increasing user engagement and retention.
-
Scalability: The use of Flask and Gunicorn allows for easy scaling of the application as business needs grow, without requiring significant architectural changes.
-
Modern UI Framework: Integration of Tailwind CSS and Flowbite enables developers to create attractive, modern user interfaces without extensive custom CSS, potentially reducing development time and costs.
-
SEO-Friendly Structure: The template's clean HTML structure and semantic markup provide a solid foundation for search engine optimization, potentially improving the website's visibility in search results.
Technologies
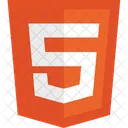