by abooooii01
AI Specific Website Scraper.
from abilities import llm_prompt
import logging
import uvicorn
from fastapi import FastAPI
from fastapi.responses import HTMLResponse
from fastapi.templating import Jinja2Templates
from selenium_utils import SeleniumUtility
# Default URL can be removed as it's now dynamically set by user input
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
app = FastAPI()
templates = Jinja2Templates(directory="templates")
from fastapi import Request, Form, Depends
# Route for the index page
# Route for the index page
@app.get("/", response_class=HTMLResponse)
async def read_root(request: Request):
return templates.TemplateResponse("index.html", {"request": request})
Frequently Asked Questions
Offering consistent and structured output format for better decision-making Q3: What industries would benefit most from implementing this scraper?
Several industries can leverage the AI Specific Website Scraper effectively: - Market research firms requiring large-scale data collection - E-commerce businesses monitoring competitor prices and products - PR and marketing agencies tracking industry news and trends - Investment firms gathering company information - Real estate agencies collecting property listings and market data
Q4: How can I modify the template to handle pagination on websites?
A: You can extend the SeleniumUtility
class in selenium_utils.py to handle pagination. Here's an example:
```python def handle_pagination(self, content: str, base_url: str): soup = BeautifulSoup(content, 'html.parser') pagination_links = set()
# Add common pagination selectors
pagination_elements = soup.select('.pagination a, .pager a, .next a')
for link in pagination_elements:
href = link.get('href')
if href:
absolute_url = urljoin(base_url, href)
if absolute_url.startswith(('http://', 'https://')):
pagination_links.add(absolute_url)
return pagination_links
```
Then update the fetch_content endpoint to include pagination handling:
```python
Add to the while loop in fetch_content
pagination_urls = selenium_util.handle_pagination(content, current_url) urls_to_visit.extend([url for url in pagination_urls if url not in visited_urls]) ```
Q5: How can I customize the LLM response schema for specific data extraction needs?
A: The AI Specific Website Scraper's response schema can be customized in the main.py file. Here's an example for extracting product information:
```python response_schema = { "type": "object", "properties": { "product_name": {"type": "string"}, "price": {"type": "number"}, "specifications": { "type": "array", "items": {"type": "string"} }, "availability": {"type": "boolean"}, "reviews": { "type": "array", "items": { "type": "object", "properties": { "rating": {"type": "number"}, "comment": {"type": "string"} } } } }, "required": ["product_name", "price", "specifications"] }
Update the llm call with new schema
extracted_info = llm( prompt=f"Extract product information from the following content:\n\n{combined_content}", model="gpt-4", temperature=0.7, response_schema=response_schema ) ```
Created: | Last Updated:
How to Use the AI Specific Website Scraper Template
This template creates a web application that can scrape and analyze specific types of information from websites using AI. The app allows you to input a URL and specify what type of information you want to extract, then provides a comprehensive analysis of the content.
Getting Started
- Click "Start with this Template" to begin using the template in Lazy Builder
Testing the Application
- Click the "Test" button in Lazy Builder
- Lazy will deploy the application and provide you with a server link to access the web interface
Using the Application
Once you access the provided server link, you'll see a simple interface where you can:
- Enter a URL in the URL input field
- Specify the type of information you want to extract in the information type field (examples: "product information", "contact details", "company history")
- Click the "Fetch Content" button to start the analysis
The application will then: * Crawl the website and related pages (up to 10 pages within the same domain) * Analyze the content using AI * Present the results including: * A comprehensive summary of the extracted information * Key points from the analysis * Confidence score of the analysis * Number of pages analyzed * List of processed URLs
FastAPI Documentation
The application includes a FastAPI backend, and Lazy will provide you with a /docs
endpoint URL where you can view the complete API documentation.
This template is designed to work as a standalone web application and doesn't require any additional integration steps. Simply use the provided server link to access and use the application through your web browser.
Template Benefits
- Automated Competitive Intelligence
- Efficiently gather and analyze competitor websites for pricing, product features, and market positioning
- Save countless hours of manual research and monitoring
-
Receive AI-analyzed summaries of competitive insights with confidence scores
-
Enhanced Market Research
- Quickly extract and analyze specific information from multiple pages within target websites
- Generate comprehensive summaries of industry trends and market data
-
Scale research capabilities without increasing headcount
-
Lead Generation & Sales Intelligence
- Extract valuable prospect information from company websites and LinkedIn profiles
- Gather detailed company information for sales outreach and qualification
-
Automate the collection of business intelligence for sales teams
-
Content Aggregation & Analysis
- Automatically collect and summarize content from multiple web pages
- Generate insights and key points from large volumes of web content
-
Support content marketing research and competitive content analysis
-
Compliance & Risk Monitoring
- Monitor websites for regulatory compliance issues
- Track changes in competitor policies and terms of service
- Generate automated reports with confidence scores for compliance teams
Technologies
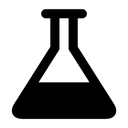
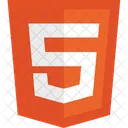


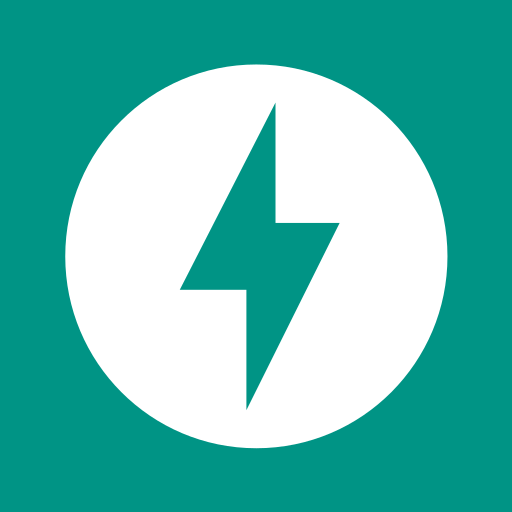

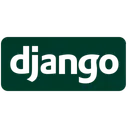