AI Discord Bot Interface
import os
import discord
from discord import app_commands
from abilities import apply_sqlite_migrations, llm
from models import Base, engine
def generate_oauth_link(client_id):
base_url = "https://discord.com/api/oauth2/authorize"
redirect_uri = "http://localhost"
scope = "bot"
permissions = "8" # Administrator permission for simplicity, adjust as needed.
return f"{base_url}?client_id={client_id}&permissions={permissions}&scope={scope}"
# Set up intents with all privileged intents enabled
intents = discord.Intents.all()
bot = discord.Client(intents=intents, activity=discord.CustomActivity("AI Chat Assistant"))
tree = app_commands.CommandTree(bot)
@bot.event
async def on_ready():
print(f'Bot is ready. Logged in as {bot.user}')
await tree.sync()
Frequently Asked Questions
How can this AI Discord Bot Interface benefit my business or community?
The AI Discord Bot Interface can significantly enhance engagement and support in your Discord server. It provides instant, contextual responses to user queries, which can be invaluable for customer service, community management, or internal team communication. For businesses, it can act as a 24/7 assistant, answering frequently asked questions, providing product information, or even assisting with basic troubleshooting. For communities, it can help moderate discussions, provide information about community rules or events, and create a more interactive environment.
What kind of customization options are available for the AI responses?
The AI Discord Bot Interface offers flexibility in customizing responses. You can adjust the 'temperature' parameter in the LLM function call to control the creativity of the responses. Additionally, you can modify the prompt given to the AI to better suit your specific use case. For example, if you're using it for a technical support channel, you could change the prompt to:
python
prompt=f"You are a technical support AI assistant in a Discord chat. Provide a helpful, technical response to this query: {content}"
This would guide the AI to give more technical, support-oriented responses.
How scalable is this AI Discord Bot Interface for larger servers or multiple servers?
The AI Discord Bot Interface is designed to be scalable. It uses Discord's official API through the discord.py library, which is capable of handling large servers and multiple servers simultaneously. The bot uses asynchronous programming, allowing it to handle multiple requests concurrently. However, for very large-scale deployments, you might need to consider factors like rate limiting from the AI service you're using and potentially implement caching or load balancing mechanisms.
How can I add more commands to the AI Discord Bot Interface?
Adding new commands to the AI Discord Bot Interface is straightforward. You can use the @tree.command()
decorator to create new slash commands. Here's an example of how you could add a simple command to get the current time:
```python from datetime import datetime
@tree.command(name='time') async def time_command(interaction): """Get the current time""" current_time = datetime.now().strftime("%H:%M:%S") await interaction.response.send_message(f"The current time is {current_time}") ```
This would add a /time
command that responds with the current time when used.
How does the AI Discord Bot Interface handle database operations?
The AI Discord Bot Interface uses SQLAlchemy for database operations, providing a powerful and flexible ORM (Object-Relational Mapping) system. It's set up to use SQLite by default, but can be easily adapted to other databases. The template includes a migration system in the main()
function:
python
def main():
apply_sqlite_migrations(engine, Base, 'migrations')
# ... rest of the function
To add new models, you would define them in models.py
using SQLAlchemy's declarative syntax. For example:
```python from sqlalchemy import Column, Integer, String from models import Base
class User(Base): tablename = 'users' id = Column(Integer, primary_key=True) name = Column(String(50)) email = Column(String(120), unique=True) ```
Then, create a new migration file in the migrations
folder to add this table to your database. The AI Discord Bot Interface will automatically apply these migrations when it starts up.
Created: | Last Updated:
Here's a step-by-step guide on how to use the AI Discord Bot Interface template:
Introduction
This template provides an AI-powered Discord bot that generates contextual responses to messages in a server. The bot uses a language model to understand and respond to user messages, making it a versatile and interactive addition to your Discord server.
Getting Started
To begin using this template, follow these steps:
- Click the "Start with this Template" button in the Lazy Builder interface.
Initial Setup
Before running the bot, you need to set up some environment secrets:
- Go to the Discord Developer Portal (https://discord.com/developers/applications).
- Create a new application and navigate to the 'Bot' section.
- Click 'Add Bot' and confirm by clicking 'Yes, do it!'.
- Under the 'TOKEN' section, click 'Copy' to get your BOT_TOKEN.
- Navigate to the 'OAuth2' section, under 'CLIENT ID', click 'Copy' to get your CLIENT_ID.
- In the Lazy Builder, go to the Environment Secrets tab.
- Add two new secrets:
- Key:
CLIENT_ID
, Value: [Your Discord Client ID] - Key:
BOT_TOKEN
, Value: [Your Discord Bot Token] - In the Discord Developer Portal, under the 'Bot' section, enable the 'Message Content Intent' slider.
Test the Bot
- Click the "Test" button in the Lazy Builder interface to start the deployment process.
- The Lazy CLI will display a link to invite your bot to a Discord server. It will look something like this:
🔗 Click this link to invite your Discord bot to your server 👉 [OAuth Link]
- Open this link in your web browser and select the Discord server where you want to add the bot.
- Grant the necessary permissions for the bot to function in your server.
Using the Bot
Once the bot is added to your server and running, you can interact with it as follows:
- Mention the bot in any channel it has access to. For example:
@YourBotName Hello!
- The bot will process your message and generate a response using its AI capabilities.
- The bot will reply to your message with its generated response.
You can also use the /echo
command:
- Type
/echo
followed by any text. - The bot will repeat the text you entered.
Integrating the Bot
This Discord bot is designed to work within Discord servers and doesn't require additional external integration. However, you can customize its behavior by modifying the code in the Lazy Builder:
- Adjust the bot's activity message in the
main.py
file. - Modify the LLM prompt in the
on_message
event handler to change how the bot responds to messages. - Add new commands using the
@tree.command
decorator.
Remember to click the "Test" button after making any changes to deploy the updated version of your bot.
By following these steps, you'll have a functional AI-powered Discord bot that can interact with users in your server. Feel free to experiment with different prompts and commands to tailor the bot to your specific needs.
Here are 5 key business benefits for this AI Discord Bot Interface template:
Template Benefits
-
Enhanced Customer Support: Businesses can deploy this bot to provide 24/7 automated customer support in their Discord communities, answering frequently asked questions and handling basic inquiries without human intervention.
-
Improved Community Engagement: The AI-powered bot can generate contextual responses to user messages, fostering more engaging and interactive conversations within Discord servers, which can lead to increased user retention and community growth.
-
Scalable Information Dissemination: Companies can use this bot to efficiently distribute information about products, services, or company updates to a large audience on Discord, ensuring consistent and accurate messaging.
-
Data Collection and Insights: By interacting with users, the bot can gather valuable data on user preferences, common questions, and pain points, providing businesses with insights to improve their products or services.
-
Cost-Effective Operations: Implementing this AI bot can reduce the need for human moderators or support staff, allowing businesses to allocate resources more efficiently while maintaining a high level of responsiveness in their Discord communities.
Technologies
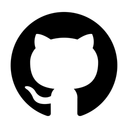

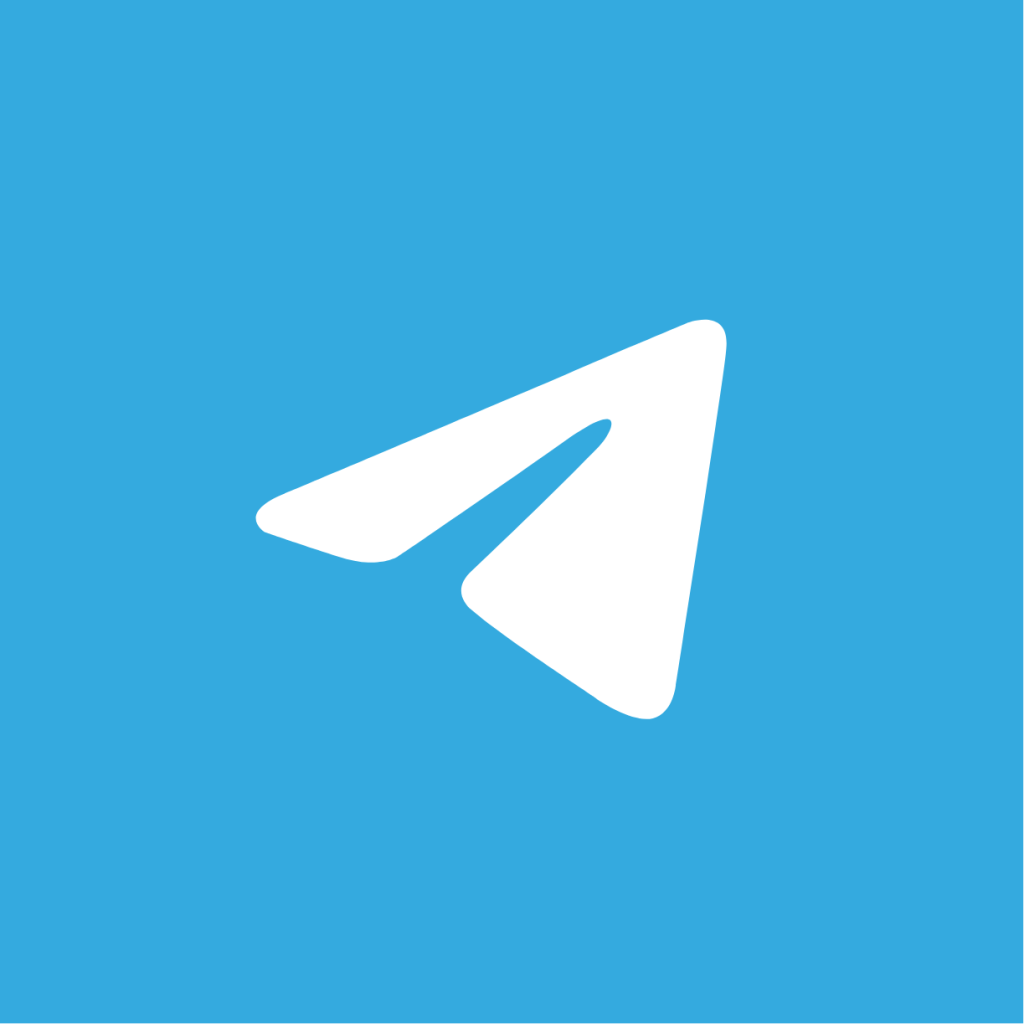
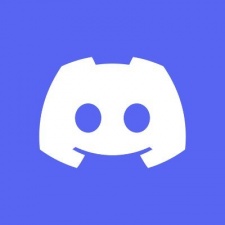