by borsacheck
Admin Panel Creator
import logging
from gunicorn.app.base import BaseApplication
from app_init import create_initialized_flask_app
# Setup logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
# Flask app creation should be done by create_initialized_flask_app to avoid circular dependency problems.
app = create_initialized_flask_app()
class StandaloneApplication(BaseApplication):
def __init__(self, app, options=None):
self.application = app
self.options = options or {}
super().__init__()
def load_config(self):
# Apply configuration to Gunicorn
for key, value in self.options.items():
if key in self.cfg.settings and value is not None:
self.cfg.set(key.lower(), value)
def load(self):
Frequently Asked Questions
What types of businesses could benefit from using the Admin Panel Creator?
The Admin Panel Creator is versatile and can benefit various businesses, including: - E-commerce platforms managing product inventories - Content management systems for blogs or news sites - Event planning companies organizing multiple events - HR departments managing employee records - Educational institutions tracking courses and student information
The template's flexibility allows it to be adapted for any business that needs to manage and organize structured data through a user-friendly interface.
How can the Admin Panel Creator improve operational efficiency?
The Admin Panel Creator can significantly enhance operational efficiency by: - Providing a centralized platform for data management - Offering an intuitive interface for creating and editing entries - Enabling quick access to information through the home page listing - Reducing the need for technical knowledge to manage data - Allowing for easy expansion of functionality as business needs grow
By streamlining data management processes, businesses can save time and reduce errors, leading to improved productivity and decision-making.
Can the Admin Panel Creator be customized for specific industry needs?
Yes, the Admin Panel Creator is designed to be highly customizable. While it comes with a basic structure for managing items with title, description, category, status, and date fields, it can be easily adapted to suit specific industry needs. For example: - A real estate company could modify the fields to include property details like square footage, number of bedrooms, and location - A library could adapt it to manage books with fields for ISBN, author, and borrowing status - A project management tool could add fields for deadlines, assigned team members, and project priorities
The template's modular structure allows for straightforward customization of both the backend data model and the frontend user interface.
How can I add custom validation to the form fields in the Admin Panel Creator?
You can add custom validation to the form fields by modifying the route handlers in the routes.py
file. Here's an example of how you might add validation for the title field to ensure it's at least 5 characters long:
```python from flask import flash
@app.route("/create", methods=['GET', 'POST']) def create_route(): if request.method == 'POST': title = request.form['title'] if len(title) < 5: flash('Title must be at least 5 characters long', 'error') return render_template("create.html"), 400 # Rest of the creation logic... ```
You can add similar validation checks for other fields and customize the error messages as needed. Remember to update the HTML templates to display these flash messages to the user.
How can I extend the Admin Panel Creator to include user authentication?
To add user authentication to the Admin Panel Creator, you can use Flask-Login, a popular extension for managing user sessions. Here's a basic example of how you might implement this:
First, install Flask-Login:
pip install flask-login
Then, update your models.py
to include a User model:
```python
from flask_login import UserMixin
class User(UserMixin, db.Model): id = db.Column(db.Integer, primary_key=True) username = db.Column(db.String(80), unique=True, nullable=False) password = db.Column(db.String(120), nullable=False) ```
In your app_init.py
, initialize Flask-Login:
```python
from flask_login import LoginManager
login_manager = LoginManager() login_manager.init_app(app)
@login_manager.user_loader def load_user(user_id): return User.query.get(int(user_id)) ```
Finally, update your routes to require login: ```python from flask_login import login_required
@app.route("/create", methods=['GET', 'POST']) @login_required def create_route(): # Your existing create route logic here ```
This basic setup will require users to log in before accessing protected routes in the Admin Panel Creator. You'll also need to create login and logout routes, as well as templates for these functions.
Created: | Last Updated:
Here's a step-by-step guide for using the Admin Panel Creator template:
Introduction
The Admin Panel Creator template provides a web-based admin panel with create and edit functionality. It features a form for data input with multiple fields and basic validation, storing data in a SQLite database. This template is ideal for quickly setting up a simple admin interface for managing items.
Getting Started
To begin using this template:
- Click the "Start with this Template" button in the Lazy Builder interface.
Test the Application
Once you've started with the template:
- Click the "Test" button in the Lazy Builder interface.
- This will initiate the deployment process and launch the Lazy CLI.
Using the Admin Panel
After the deployment is complete, you'll receive a dedicated server link to access your admin panel. Here's how to use it:
- Open the provided link in your web browser.
- You'll see the home page of your admin panel, displaying existing items (if any).
- To create a new item:
- Click the "Create New Item" button.
- Fill out the form with the item's details (title, description, category, status, and date).
- Click "Submit" to add the new item.
- To edit an existing item:
- Click the "Edit" button next to the item you want to modify.
- Update the item's details in the form.
- Click "Update Item" to save your changes.
Customizing the Admin Panel
You can customize the admin panel by modifying the following files in the Lazy Builder interface:
models.py
: Adjust theItem
model to change the database schema.routes.py
: Modify or add new routes to extend functionality.home.html
,create.html
, andedit.html
: Update the HTML templates to change the UI.styles.css
: Modify the CSS to adjust the appearance of the admin panel.
Remember to test your changes by clicking the "Test" button after making modifications.
By following these steps, you'll have a functional admin panel up and running, ready for managing your items through a user-friendly web interface.
Here are 5 key business benefits for this Admin Panel Creator template:
Template Benefits
-
Rapid Prototyping: This template allows businesses to quickly set up a functional admin panel, enabling faster development of internal tools and management systems.
-
Customizable Data Management: The flexible item model and form structure make it easy to adapt the panel for various business needs, from inventory management to customer data handling.
-
User-Friendly Interface: With a responsive design and intuitive navigation, this template provides a smooth user experience for staff members, reducing training time and improving productivity.
-
Scalable Architecture: Built on Flask with SQLAlchemy, the template offers a solid foundation that can be easily expanded to accommodate growing business needs and additional features.
-
Cost-Effective Solution: By providing a ready-to-use admin panel structure, this template significantly reduces development time and costs associated with building custom administrative tools from scratch.
Technologies
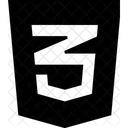
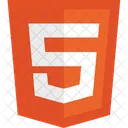