by eliaschow1
URL Text Extractor with BeautifulSoup
import logging
from gunicorn.app.base import BaseApplication
from app_init import create_initialized_flask_app
# Flask app creation should be done by create_initialized_flask_app to avoid circular dependency problems.
app = create_initialized_flask_app()
# Setup logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
class StandaloneApplication(BaseApplication):
def __init__(self, app, options=None):
self.application = app
self.options = options or {}
super().__init__()
def load_config(self):
# Apply configuration to Gunicorn
for key, value in self.options.items():
if key in self.cfg.settings and value is not None:
self.cfg.set(key.lower(), value)
def load(self):
Created: | Last Updated:
Here's a step-by-step guide for using the URL Text Extractor with BeautifulSoup template:
Introduction
The URL Text Extractor with BeautifulSoup template provides a web application that allows users to extract and display text content from a given URL using BeautifulSoup. This tool is useful for quickly retrieving the main text content from web pages without the need to manually copy and paste.
Getting Started
- Click "Start with this Template" to begin using the URL Text Extractor template in the Lazy Builder interface.
Test the Application
-
Press the "Test" button in the Lazy Builder interface to deploy and launch the application.
-
Once the deployment is complete, Lazy will provide you with a dedicated server link to access the web application.
Using the App
-
Open the provided server link in your web browser to access the URL Text Extractor interface.
-
You'll see a simple form with an input field and a "Extract Text" button.
-
Enter the URL of the web page you want to extract text from in the input field.
-
Click the "Extract Text" button to initiate the text extraction process.
-
The application will fetch the content from the provided URL, extract the text using BeautifulSoup, and display the results on the same page.
-
If successful, you'll see the extracted text displayed in a scrollable area below the form.
-
If there's an error (e.g., invalid URL or connection issues), an error message will be displayed instead.
-
You can repeat the process with different URLs as needed.
Notes
- The application uses BeautifulSoup to parse HTML content and extract text, which helps remove most HTML tags and formatting.
- The extracted text is displayed with line breaks to improve readability.
- The interface is responsive and works on both desktop and mobile devices.
By following these steps, you'll be able to use the URL Text Extractor with BeautifulSoup template to easily extract and view text content from various web pages.
Technologies
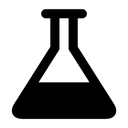
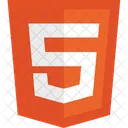
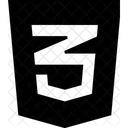