by Barandev
Stripe API Testing using Flask
from flask import Flask, render_template, request, jsonify
import os
import requests
import stripe
app = Flask(__name__)
# Set up environment variables for Stripe API keys
# stripe.api_key is now set within the update_info function using the user-provided API secret
# Base URL for Stripe API
stripe_base_url = 'https://api.stripe.com/v1'
# Define the variable for the test customer ID
TEST_CUSTOMER_ID = os.environ.get('TEST_CUSTOMER_ID', None)
@app.route('/')
def index():
return render_template('template.html')
@app.route('/update_info', methods=['POST'])
def update_info():
api_secret = request.form['apiSecret']
customer_id = request.form.get('customerId') # Get customerId if provided by user
Frequently Asked Questions
How can businesses benefit from using this Stripe API Testing template?
The Stripe API Testing template offers several benefits for businesses: - It allows quick validation of Stripe integration, ensuring smooth payment processing. - Businesses can test critical functions like adding cards and creating charges without affecting live data. - It helps in identifying potential issues early in the development process, saving time and resources. - The template can be used for ongoing testing to ensure continued functionality after updates or changes to the payment system.
Is this Stripe API Testing template suitable for small businesses or startups?
Yes, the Stripe API Testing template is particularly valuable for small businesses and startups. It provides a simple, cost-effective way to test Stripe integration without the need for extensive development resources. The template's user-friendly interface allows even non-technical team members to run basic tests, making it an accessible tool for businesses at any stage of growth.
How does the Stripe API Testing template ensure security when handling sensitive payment information?
The Stripe API Testing template prioritizes security in several ways: - It uses environment variables to store sensitive information like API keys. - The template doesn't store any payment information locally. - It leverages Stripe's secure API for all transactions. - The use of test tokens (like 'tok_visa') ensures no real card data is used during testing.
How can I modify the Stripe API Testing template to include additional test cases?
You can easily extend the run_stripe_tests
function in the main.py
file to include additional test cases. For example, to add a test for creating a subscription, you could add the following code:
```python def run_stripe_tests(api_secret, customer_id): test_results = {} # ... existing tests ...
# Test creating a subscription
plan_id = 'plan_example' # Replace with your actual plan ID
subscription_result = create_subscription(customer_id, plan_id)
test_results['SubscriptionCreated'] = subscription_result.get('id') is not None
return test_results
def create_subscription(customer_id, plan_id): try: subscription = stripe.Subscription.create( customer=customer_id, items=[{'plan': plan_id}] ) return subscription except stripe.error.StripeError as e: print(f"Error creating subscription: {e}") return None ```
Remember to update the frontend to display the new test result as well.
How can I customize the error handling in the Stripe API Testing template?
The template uses basic error handling, but you can enhance it by implementing more detailed error logging and user-friendly error messages. Here's an example of how you could modify the add_card_to_customer
function:
```python from logging_config import configure_logging import logging
configure_logging() logger = logging.getLogger(name)
def add_card_to_customer(customer_id, card_token): try: card = stripe.Customer.create_source( customer_id, source=card_token ) logger.info(f"Successfully added card {card.id} to customer {customer_id}") return card.id except stripe.error.CardError as e: logger.error(f"Card error for customer {customer_id}: {e.user_message}") return {"error": "Invalid card details. Please check and try again."} except stripe.error.StripeError as e: logger.error(f"Stripe error for customer {customer_id}: {str(e)}") return {"error": "An unexpected error occurred. Please try again later."} ```
This modification adds more specific error handling and logging, which can help in debugging and providing more informative feedback to users of the Stripe API Testing template.
Created: | Last Updated:
Introduction to the Stripe API Integration Tester Template
Welcome to the Stripe API Integration Tester Template! This template is designed to help you test Stripe API integrations with ease. It provides a web-based interface where you can input your Stripe API secret and optionally a customer ID to run a series of tests, such as adding a card to a customer and creating a charge. This step-by-step guide will walk you through using the template on the Lazy platform.
Getting Started
To begin using this template, simply click on "Start with this Template" on the Lazy platform. This will pre-populate the code in the Lazy Builder interface, so you won't need to copy, paste, or delete any code.
Initial Setup
Before you can test the Stripe API, you'll need to set up an environment secret for the test customer ID. Here's how to do it:
- Log in to your Stripe dashboard and navigate to the "Customers" section.
- Create a new customer or use an existing one and copy the customer ID.
- Go to the Environment Secrets tab within the Lazy Builder.
- Create a new secret with the key
TEST_CUSTOMER_ID
and paste the customer ID as the value.
Test: Pressing the Test Button
Once you have set up the environment secret, press the "Test" button on the Lazy platform. This will begin the deployment of the app and launch the Lazy CLI.
Entering Input
After pressing the "Test" button, the Lazy App's CLI interface will appear, prompting you to provide the Stripe API secret. You can obtain your API secret key from your Stripe dashboard under the "Developers" section. Copy the secret key and paste it into the CLI when prompted.
Using the App
After providing the necessary input, Lazy will print a dedicated server link for you to use the API. Navigate to this link in your web browser to access the Stripe API Integration Tester interface.
Here's how to use the interface:
- Enter your Stripe API secret into the "API Secret" field.
- If you have a specific customer ID you want to use, enter it into the "Customer ID" field. Otherwise, leave it blank, and a test customer will be created for you.
- Click the "Run Tests" button to execute the tests.
- The test results will be displayed on the page, indicating whether each test passed or failed.
Integrating the App
If you wish to integrate this testing functionality into another service or frontend, you can use the provided endpoints and JavaScript code. For example, you can send a POST request to the /update_info
endpoint with the API secret and customer ID to run the tests programmatically.
Here's a sample request you might use:
`POST /update_info
Content-Type: application/x-www-form-urlencoded
apiSecret=your_stripe_api_secret&customerId=optional_customer_id` And a sample response could look like this:
{
"CardAdded": true,
"ChargeCreated": true,
"CustomerDetailsRetrieved": true
}
Remember to replace your_stripe_api_secret
with your actual Stripe API secret and optional_customer_id
with the customer ID you wish to test with, if any.
By following these steps, you can easily test and integrate Stripe API functionality using the Lazy platform.
Here are 5 key business benefits for this Stripe API testing template:
Template Benefits
-
Rapid API Integration Testing: Enables developers to quickly test and verify Stripe API functionality, accelerating the integration process for payment systems.
-
Custom Payment Flow Validation: Allows businesses to validate custom payment flows and ensure they work correctly with Stripe before deploying to production.
-
Developer Onboarding Tool: Serves as an excellent onboarding tool for new developers joining a project, helping them understand and test Stripe integrations easily.
-
Troubleshooting Aid: Provides a simple interface for isolating and diagnosing issues with Stripe API calls, streamlining the debugging process.
-
Compliance Verification: Helps businesses verify that their Stripe integration meets compliance requirements by allowing easy testing of key payment processes.
Technologies
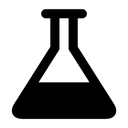
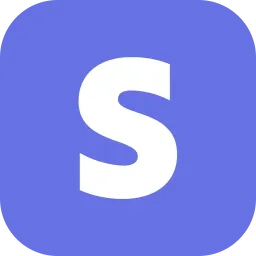