by Lazy Sloth
Login and Registration
from forms import AccountForm
import logging
from flask import session
from flask import render_template, request, redirect, url_for, flash
from app_init import app
from models import db, User
from forms import LoginForm, RegistrationForm
# Removed Flask app initialization here. It will be initialized in app_init.py and imported.
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///users.db'
app.config['SECRET_KEY'] = 'a_very_secret_key'
db.init_app(app)
with app.app_context():
db.create_all()
@app.route("/account", methods=['GET', 'POST'])
def account():
if 'username' not in session:
flash('You must be logged in to view this page.')
return redirect(url_for('login'))
form = AccountForm()
Login and Registration
Created: | Last Updated:
Introduction to the Login and Registration Template
This template provides a robust starting point for building applications that require user authentication, including features for user registration, login, and account management. It leverages Flask, a micro web framework written in Python, to handle backend operations, and uses SQLAlchemy for database interactions. The frontend is styled with Tailwind CSS for a modern and responsive design.
Getting Started with the Template
To begin using this template, click on "Start with this Template" in the Lazy builder interface. This action will set up the template in your Lazy workspace, allowing you to customize and deploy your application without worrying about environment setup or library installations.
Test: Deploying the App
Once you have the template in your workspace, you can deploy your application by clicking the "Test" button. Lazy will handle the deployment process, and you will not need to install any dependencies manually.
Entering Input
If the template requires user input through the CLI, you will be prompted to provide this information after pressing the "Test" button. Follow the instructions in the Lazy CLI to enter the necessary details.
Using the App
After deployment, you will be provided with a server link to access your application. Navigate to this link to interact with the user interface. Here's what you can do:
- Register a new user account by filling out the registration form with a username, email, and password.
- Login with an existing user account using either the username or email and password.
- Access account settings to update the username or password after logging in.
- Logout from the application, which will redirect you to the home page.
The home page will display a welcome message and provide options to register or login depending on the user's authentication status.
Integrating the App
If you wish to integrate this application with other services or tools, you may need to provide the server link generated by Lazy to those external platforms. For example, if you want to use this authentication system within another web application, you would include the server link as the endpoint for login and registration actions.
Additionally, if your integration requires API endpoints, you can use the provided server link to make HTTP requests to the login, registration, and account management endpoints defined in the Flask application.
Remember, all the necessary steps to deploy and run your application are handled by Lazy, so you can focus on building and integrating your app with ease.
Technologies

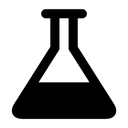