by Lazy Sloth
Login and Registration
import logging
from flask import Flask
from gunicorn.app.base import BaseApplication
from routes import register_routes
from models import db
from migrations import run_migrations
def create_app():
app = Flask(__name__, static_folder='static')
app.secret_key = 'supersecretkey'
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///database.sqlite'
db.init_app(app)
with app.app_context():
db.create_all()
run_migrations(app)
register_routes(app)
return app
app = create_app()
# Setup logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
Login and Registration
Created: | Last Updated:
Introduction to the Login and Registration Template
This template provides a starting point for any application that requires user login and registration functionality. It includes pre-built HTML templates, CSS for styling, JavaScript for interactivity, and Python code for backend operations using Flask. The template handles user registration, login, and logout processes, and includes a responsive navigation menu for both mobile and desktop views.
Clicking Start with this Template
To get started with the template, click the "Start with this Template" button in the Lazy Builder interface.
Test
Press the "Test" button in the Lazy Builder interface to deploy the app. This will launch the Lazy CLI, and you will be prompted for any required user input.
Using the App
Once the app is deployed, you can interact with it through the provided interface. The app includes the following pages:
- Home Page: Displays login and registration options if the user is not logged in. If the user is logged in, it shows a message indicating that the user is logged in.
- Register Page: Allows new users to register by providing an email and password.
- Login Page: Allows existing users to log in by providing their email and password.
Home Page
The home page is accessible at the root URL ("/"). It displays login and registration options if the user is not logged in. If the user is logged in, it shows a message indicating that the user is logged in.
Register Page
The register page is accessible at the "/register" URL. It allows new users to register by providing an email and password. Upon successful registration, the user is redirected to the login page.
Login Page
The login page is accessible at the "/login" URL. It allows existing users to log in by providing their email and password. Upon successful login, the user is redirected to the home page.
Integrating the App
If you need to integrate this app with other tools or services, follow these steps:
- API Integration: If your app requires API endpoints, you can use the provided server link to interact with the app. For example, you can use the login and registration endpoints to authenticate users in your external tool.
- Frontend Integration: If you need to integrate the app's frontend components into another tool, you can use the provided HTML templates and CSS styles. Simply copy the relevant code and include it in your external tool's frontend.
Sample API Request and Response
If your app includes API endpoints, here is a sample request and response for the login endpoint:
`POST /login
Content-Type: application/json
{
"email": "user@example.com",
"password": "password123"
}HTTP/1.1 200 OK
Content-Type: application/json
{
"message": "Login successful",
"user_id": 1,
"user_email": "user@example.com"
}`
By following these steps, you can successfully deploy and integrate the Login and Registration template into your application. If you have any questions or need further assistance, please refer to the Lazy documentation or contact Lazy support.
Technologies
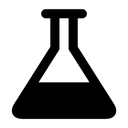
