by Lazy Sloth
Get All Events by Date in Google Calendar API
import os
import logging
from fastapi import FastAPI, HTTPException
from fastapi.responses import HTMLResponse, JSONResponse
from pydantic import BaseModel
import requests
from datetime import datetime
logger = logging.getLogger(__name__)
logging.basicConfig(level=logging.WARNING)
app = FastAPI()
# The builder needs to provide the Google Calendar API key in the Env Secrets tab.
GOOGLE_CALENDAR_API_KEY = os.environ.get('GOOGLE_CALENDAR_API_KEY')
if not GOOGLE_CALENDAR_API_KEY:
raise Exception("The GOOGLE_CALENDAR_API_KEY environment variable is not set.")
def fetch_events(calendar_id: str, date: str):
url = f"https://www.googleapis.com/calendar/v3/calendars/{calendar_id}/events"
params = {
'key': GOOGLE_CALENDAR_API_KEY,
'timeMin': f"{date}T00:00:00Z",
'timeMax': f"{date}T23:59:59Z",
Frequently Asked Questions
How can businesses benefit from using this Google Calendar Events by Date application?
The Get All Events by Date in Google Calendar API application offers several benefits for businesses: - Efficient event management: Quickly retrieve and view all events for a specific date. - Integration possibilities: Easily incorporate calendar data into other business systems or applications. - Improved scheduling: Make informed decisions about resource allocation and time management. - Event analysis: Download event data in JSON format for further analysis or reporting.
Can this application be used for multiple Google Calendars within an organization?
Yes, the Get All Events by Date in Google Calendar API application can be used with multiple calendars. Each calendar has a unique Calendar ID, which can be entered when fetching events. This allows organizations to manage and retrieve events from various department or project-specific calendars as needed.
How can marketing teams utilize this application for event planning and promotion?
Marketing teams can leverage this application in several ways: - Event coordination: Easily view all marketing events scheduled for a particular date. - Content planning: Use the retrieved event data to plan social media posts or email campaigns. - Avoid conflicts: Ensure new marketing initiatives don't clash with existing events. - Performance tracking: Download event data to analyze attendance or engagement metrics over time.
How can I modify the application to include additional event details in the response?
To include additional event details, you can modify the fetch_events
function in the application. The Google Calendar API returns various fields for each event, which you can include in your response. Here's an example of how you might modify the function to include the event location and attendees:
python
def fetch_events(calendar_id: str, date: str):
# ... existing code ...
events_data = response.json().get('items', [])
formatted_events = []
for event in events_data:
formatted_event = {
'summary': event.get('summary'),
'start': event.get('start'),
'end': event.get('end'),
'description': event.get('description'),
'location': event.get('location'),
'attendees': [attendee.get('email') for attendee in event.get('attendees', [])]
}
formatted_events.append(formatted_event)
return formatted_events
This modification will include the event location and a list of attendee emails in the response.
Is it possible to add authentication to the Get All Events by Date in Google Calendar API application?
Yes, you can add authentication to secure the application. One way to do this is by using FastAPI's built-in security utilities. Here's an example of how you could add basic HTTP authentication:
```python from fastapi import Depends, HTTPException, status from fastapi.security import HTTPBasic, HTTPBasicCredentials import secrets
security = HTTPBasic()
def authenticate(credentials: HTTPBasicCredentials = Depends(security)): correct_username = secrets.compare_digest(credentials.username, "admin") correct_password = secrets.compare_digest(credentials.password, "password") if not (correct_username and correct_password): raise HTTPException( status_code=status.HTTP_401_UNAUTHORIZED, detail="Incorrect email or password", headers={"WWW-Authenticate": "Basic"}, ) return credentials.username
@app.get("/events/{calendar_id}/{date}") def get_events(calendar_id: str, date: str, username: str = Depends(authenticate)): # ... existing code ... ```
This modification adds basic authentication to the /events
endpoint. Users will need to provide a username and password to access the events data. Remember to use more secure authentication methods and store credentials safely in a production environment.
Created: | Last Updated:
Introduction to the Google Calendar Events by Date Template
Welcome to the Lazy template guide for retrieving events from a Google Calendar on a specific date. This template is designed to help you build an application that interacts with the Google Calendar API to fetch and display events for a given date. It includes endpoints to get events in a list format and to download them as a JSON file. Additionally, it provides a simple HTML frontend to interact with these endpoints.
Getting Started
To begin using this template, click on "Start with this Template" in the Lazy builder interface. This will pre-populate the code in the Lazy Builder, so you won't need to copy or paste any code manually.
Initial Setup
Before you can use the application, you need to set up an environment secret for the Google Calendar API key:
- Go to the Google Developers Console (https://console.developers.google.com/).
- Create a new project or select an existing one.
- Enable the Google Calendar API for your project.
- Create credentials (API key) for your project.
- Copy the generated API key.
- In the Lazy Builder interface, navigate to the Environment Secrets tab.
- Create a new secret with the key as
GOOGLE_CALENDAR_API_KEY
and paste your copied API key as the value.
Test
Once you have set up the environment secret, press the "Test" button. This will deploy your application and launch the Lazy CLI. The CLI will not prompt for any user input as all necessary information is provided through the API endpoints.
Using the App
After pressing the "Test" button, Lazy will provide you with a dedicated server link to access your application. If you're using FastAPI, Lazy will also provide a link to the API documentation.
To interact with the app:
- Open the provided server link in your web browser to view the simple HTML frontend.
- Enter a public Google Calendar ID and a date in the respective fields.
- Click on "Fetch Events" to display the events for the specified date.
- Click on "Download Events" to download the events as a JSON file.
Integrating the App
If you wish to integrate this application into another service or frontend, you can use the provided API endpoints. Here's an example of how to make a request to the API:
GET /events/{calendar_id}/{date}
Replace {calendar_id}
with the ID of the Google Calendar and {date}
with the date you want to fetch events for (in YYYY-MM-DD format).
A sample response from the API might look like this:
[
{
"start": {"dateTime": "2023-04-01T10:00:00Z"},
"end": {"dateTime": "2023-04-01T11:00:00Z"},
"summary": "Event Title",
"description": "Event Description"
},
// ... more events
]
Use the server link provided by Lazy to make requests to your deployed application. If you need to integrate these endpoints into a frontend application, you can make AJAX calls to these endpoints and process the response as needed.
Here are 5 key business benefits for this template:
Template Benefits
-
Efficient Event Management: Businesses can quickly retrieve and view all events scheduled for a specific date, enabling better planning and resource allocation.
-
Integration Capabilities: The template provides a foundation for integrating Google Calendar data into other business systems, such as CRM or project management tools, enhancing overall workflow efficiency.
-
Client Communication: Service-based businesses can use this to easily access and share daily schedules with clients, improving transparency and customer service.
-
Data Analysis and Reporting: The ability to download event data in JSON format allows for easy import into data analysis tools, facilitating insights into scheduling patterns and resource utilization.
-
Customizable User Interface: The included HTML interface can be easily modified to match company branding or specific business needs, providing a professional and consistent user experience.
Technologies
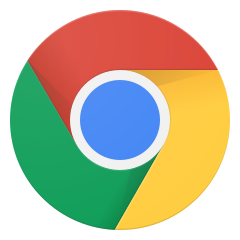