by Lazy Sloth
Discord Poll Bot
import os
import discord
from discord.ext import commands
from collections import defaultdict
import asyncio
import re
# Retrieve the Discord bot token from the environment variables
DISCORD_BOT_TOKEN = os.environ.get('DISCORD_BOT_TOKEN')
intents = discord.Intents.default()
intents.messages = True
intents.reactions = True
bot = commands.Bot(command_prefix='/', intents=intents)
class Poll:
def __init__(self, question, options, duration=24):
self.question = question + " 🤔"
self.options = options
self.votes = defaultdict(int)
self.message = None
self.duration = duration * 3600 # Convert hours to seconds
self.poll_task = None
Frequently Asked Questions
How can this Discord Poll Bot benefit my online community or business?
The Discord Poll Bot can greatly enhance engagement and decision-making in your online community or business. It allows you to quickly gather opinions on various topics, from product preferences to event planning. For businesses, it can be used to conduct market research, gauge employee satisfaction, or even make team decisions. The bot's user-friendly interface and real-time voting make it an excellent tool for fostering participation and transparency in your Discord server.
Can I customize the poll duration for time-sensitive decisions?
Absolutely! The Discord Poll Bot is designed with flexibility in mind. You can easily set custom durations for your polls, which is particularly useful for time-sensitive decisions. For example, if you're running a quick team vote during a meeting, you could set a short duration like 5 minutes. For more complex decisions that require careful consideration, you might set a longer duration of several hours or even days. This feature allows you to adapt the polling process to your specific needs and timelines.
How secure is the voting process with this Discord Poll Bot?
The Discord Poll Bot leverages Discord's built-in reaction system for voting, which provides a basic level of security. Each user can only react once per option, preventing multiple votes from a single user. However, it's important to note that this bot doesn't implement advanced anti-fraud measures. For most casual polls and community decisions, this level of security is sufficient. If you require a more secure voting system for critical decisions, you may need to consider additional verification methods or a more specialized voting platform.
How can I modify the Discord Poll Bot to support image options in polls?
To add image support to the Discord Poll Bot, you would need to modify the Poll
class and the poll
command. Here's a basic example of how you might implement this:
```python class Poll: def init(self, question, options, images, duration=24): self.question = question + " 🤔" self.options = options self.images = images # ... rest of the init method ...
async def start(self, ctx):
description = []
for emoji, option in self.options.items():
description.append(f"{emoji} : {option}")
embed = discord.Embed(title=self.question, description='\n'.join(description))
if self.images:
embed.set_image(url=self.images[0]) # Set the first image
self.message = await ctx.send(embed=embed)
# ... rest of the start method ...
@bot.command(name='poll') async def poll(ctx, *, message: str): # ... existing code ... options_str = match.group(4) images_str = match.group(5) # New group for images
options = [option.strip(' "') for option in options_str.split(',')]
images = [image.strip() for image in images_str.split(',')] if images_str else []
poll = Poll(question, poll_options, images, duration)
# ... rest of the command ...
```
This modification allows users to include image URLs with their poll options, enhancing the visual appeal of the polls.
How can I implement a feature to allow users to end polls early?
To add an early poll ending feature to the Discord Poll Bot, you can create a new command and modify the Poll
class. Here's an example implementation:
```python @bot.command(name='endpoll') async def end_poll(ctx, poll_id: int): if poll_id in polls: poll = polls[poll_id] if ctx.author == poll.author: # Check if the command user is the poll creator await poll.close() await ctx.send(f"Poll {poll_id} has been closed early.") else: await ctx.send("You don't have permission to end this poll.") else: await ctx.send("Poll not found.")
class Poll: def init(self, question, options, duration=24, author=None): self.author = author # ... rest of the init method ...
async def start(self, ctx):
self.author = ctx.author # Store the poll creator
# ... rest of the start method ...
```
This implementation adds an endpoll
command that allows the original poll creator to end the poll early. It also modifies the Poll
class to store the author of each poll. This feature gives users more control over their polls in the Discord Poll Bot, allowing for greater flexibility in managing discussions and decision-making processes.
Created: | Last Updated:
Introduction to the Discord Poll Bot Template
Welcome to the Discord Poll Bot template! This template allows you to create a Discord bot that can facilitate polls within your Discord server. Users can interact with the bot using the "/poll" command to create polls with custom questions, options, and durations. The bot will then present the poll with reaction emojis for voting and tally the votes in real-time, announcing the winning option when the poll closes.
Getting Started with the Template
To begin using this template, simply click on "Start with this Template" on the Lazy platform. This will pre-populate the code in the Lazy Builder interface, so you won't need to copy, paste, or delete any code.
Initial Setup: Adding Environment Secrets
Before you can test and use the Discord Poll Bot, you need to set up an environment secret for the Discord bot token. Here's how to acquire and set up your Discord bot token:
- Go to the Discord Developer Portal and create a new application.
- Inside your application, navigate to the "Bot" tab and create a new bot.
- Under the bot settings, you will find the token. Copy this token as you will need it for the next step.
- In the Lazy Builder interface, go to the Environment Secrets tab.
- Create a new secret with the key 'DISCORD_BOT_TOKEN' and paste the token you copied as the value.
Test: Pressing the Test Button
Once you have set up your environment secret, you can press the "Test" button to begin the deployment of your Discord Poll Bot. The Lazy CLI will handle the deployment process, and you won't need to provide any additional input at this stage.
Using the App
After deployment, your Discord Poll Bot will be live on your Discord server. To create a poll, use the "/poll" command followed by the question, duration, and options. For example:
/poll question={Do you like pizza?} duration={1} options={Yes, No, Maybe}
Users can vote by reacting to the poll message with the corresponding emojis. The bot will automatically close the poll after the specified duration and announce the results.
Integrating the App
If you wish to integrate the Discord Poll Bot with other tools or services, you may need to provide the bot's server link or API endpoints. Since this template is designed to work within Discord, no additional external integration steps are required unless you plan to extend the bot's functionality beyond the provided template.
Remember, this bot is designed to work within the Lazy platform, so there's no need to worry about local environments or operating systems. Enjoy creating engaging polls for your Discord community!
Template Benefits
-
Enhanced Employee Engagement: This Discord Poll Bot can be used in company Discord servers to quickly gather employee opinions on various topics, from project decisions to office policies, fostering a culture of participation and inclusivity.
-
Streamlined Decision Making: By allowing teams to create polls easily, the bot facilitates faster and more democratic decision-making processes, especially useful for distributed or remote teams.
-
Real-time Feedback Collection: Businesses can use this bot to collect instant feedback on products, services, or ideas from customers or team members, enabling agile responses to market needs or internal suggestions.
-
Event Planning Simplification: The bot simplifies the process of organizing company events or team-building activities by allowing easy voting on dates, venues, or activity preferences.
-
Training and Development Insights: HR departments can utilize the bot to gauge interest in different training programs or to assess knowledge gaps within the organization, helping to tailor professional development initiatives more effectively.
Technologies
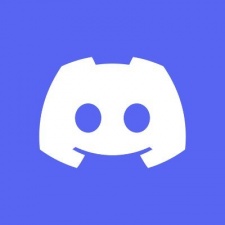