Convert PDF to CSV File Format
import logging
from fastapi import FastAPI, File, UploadFile, HTTPException
from fastapi.responses import HTMLResponse, StreamingResponse
import io
import os
import pdfplumber
import pandas as pd
# Configure logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
app = FastAPI()
# Function to convert PDF to CSV
def convert_pdf_to_csv(pdf_stream):
try:
# Load PDF
pdf = pdfplumber.open(pdf_stream)
first_page = pdf.pages[0]
table = first_page.extract_table()
pdf.close()
# Convert table to DataFrame
Frequently Asked Questions
What are some business applications for this PDF to CSV converter?
The Convert PDF to CSV File Format app has numerous business applications. It can be used in finance departments to convert PDF financial reports into CSV format for easier analysis. HR departments can use it to transform PDF resumes into structured CSV data for applicant tracking systems. Marketing teams can convert PDF survey results or market research reports into CSV for data visualization tools. Essentially, any business that deals with data trapped in PDF format can benefit from this converter.
How can this tool improve efficiency in data processing workflows?
The Convert PDF to CSV File Format app significantly improves efficiency by automating a typically manual and time-consuming process. Instead of manually retyping data from PDFs into spreadsheets, users can quickly upload a PDF and receive a structured CSV file. This not only saves time but also reduces the risk of human error in data entry. For businesses dealing with large volumes of PDF documents, this tool can lead to substantial time and cost savings.
What industries could benefit most from this PDF to CSV converter?
Several industries can greatly benefit from the Convert PDF to CSV File Format app: - Financial services: For converting financial statements and reports - Healthcare: To transform patient records or medical research data - Education: For processing student records or research papers - Real estate: To convert property listings or market reports - Legal: For processing case documents or contracts Any industry that regularly deals with data-rich PDF documents can find value in this tool.
How can I modify the code to handle multi-page PDFs?
To handle multi-page PDFs in the Convert PDF to CSV File Format app, you can modify the convert_pdf_to_csv
function. Instead of only processing the first page, you can iterate through all pages:
```python def convert_pdf_to_csv(pdf_stream): try: pdf = pdfplumber.open(pdf_stream) all_tables = [] for page in pdf.pages: table = page.extract_table() if table: all_tables.extend(table) pdf.close()
df = pd.DataFrame(all_tables[1:], columns=all_tables[0])
csv_stream = io.StringIO()
df.to_csv(csv_stream, index=False, quoting=1, encoding='utf-8')
csv_stream.seek(0)
return csv_stream
except Exception as e:
logger.error(f"Failed to convert PDF to CSV: {e}")
raise HTTPException(status_code=500, detail="Failed to convert PDF to CSV.")
```
This modification will process all pages of the PDF and combine the results into a single CSV file.
How can I add error handling for specific PDF conversion issues?
To add more specific error handling in the Convert PDF to CSV File Format app, you can modify the convert_pdf_to_csv
function to catch and handle specific exceptions. Here's an example:
```python from pdfminer.pdfpage import PDFPageCountError
def convert_pdf_to_csv(pdf_stream): try: pdf = pdfplumber.open(pdf_stream) # ... rest of the conversion code ... except PDFPageCountError: logger.error("PDF has no pages") raise HTTPException(status_code=400, detail="The uploaded PDF has no pages.") except pdfplumber.PDFSyntaxError: logger.error("Invalid PDF syntax") raise HTTPException(status_code=400, detail="The uploaded file is not a valid PDF.") except Exception as e: logger.error(f"Failed to convert PDF to CSV: {e}") raise HTTPException(status_code=500, detail="Failed to convert PDF to CSV.") ```
This modification adds specific error handling for common PDF-related issues, providing more informative error messages to the user.
Created: | Last Updated:
Introduction to the PDF to CSV Converter Template
Welcome to the Lazy template guide for converting PDF files to CSV format. This template is designed to help you quickly set up an application that allows users to upload a PDF file and receive a CSV file in return. The application uses FastAPI to create a simple web server with endpoints for uploading the PDF and downloading the converted CSV file.
Clicking Start with this Template
To begin using this template, click on the "Start with this Template" button. This will pre-populate the code in the Lazy Builder interface, so you won't need to copy, paste, or delete any code manually.
Test: Pressing the Test Button
Once you have the template loaded, press the "Test" button to deploy the application. Lazy will handle the deployment process, and you won't need to worry about installing libraries or setting up your environment.
Using the App
After pressing the "Test" button, Lazy will provide you with a dedicated server link to use the API. You will also receive a link to the FastAPI documentation, which will be useful for understanding how to interact with the API endpoints.
The application has two main endpoints:
- A POST endpoint at
/upload_pdf
for uploading the PDF file. - A GET endpoint at
/
which serves the main page with the file upload form.
To use the application:
- Go to the main page served at the root endpoint.
- Use the form to select and upload a PDF file.
- After the file is uploaded and processed, a download link will appear.
- Click on the download link to receive the converted CSV file.
The main page contains a simple HTML form where users can upload their PDF files. The JavaScript code handles the form submission and fetches the converted CSV file from the server.
Integrating the App
If you wish to integrate this application into an external service or frontend, you can use the server link provided by Lazy to make API calls from your external tool. For example, you can set up a button in your external tool that, when clicked, sends a request to the /upload_pdf
endpoint and handles the response.
Here is a sample code snippet that you could use in an external tool to integrate with the application:
`// Example JavaScript code to call the upload_pdf endpoint
async function uploadPDF(file) {
const formData = new FormData();
formData.append('file', file);
try {
const response = await fetch('YOUR_LAZY_SERVER_LINK/upload_pdf', {
method: 'POST',
body: formData,
});
if (response.ok) {
const blob = await response.blob();
const downloadUrl = URL.createObjectURL(blob);
// You can then set the downloadUrl to a download link or trigger a download directly
} else {
console.error('Error uploading PDF:', response.statusText);
}
} catch (error) {
console.error('Network error:', error);
}
}Replace
YOUR_LAZY_SERVER_LINK` with the server link provided by Lazy after deployment. This code can be added to your external tool's frontend to allow users to upload PDF files and receive CSV files in return.
Remember, this template is designed to work seamlessly on the Lazy platform, so all the heavy lifting of deployment and environment configuration is taken care of for you. Enjoy building your PDF to CSV converter application!
Here are 5 key business benefits for this PDF to CSV conversion template:
Template Benefits
-
Improved Data Accessibility: By converting PDF tables to CSV format, businesses can easily import data into various analysis tools and databases, making information more accessible and usable across different platforms.
-
Time and Cost Savings: Automating the conversion process eliminates the need for manual data entry, saving significant time and reducing labor costs associated with transferring information from PDFs to spreadsheets.
-
Enhanced Data Accuracy: The automated conversion reduces human error that can occur during manual data entry, ensuring higher accuracy in the extracted data and improving overall data quality for business operations.
-
Streamlined Workflow: With a user-friendly web interface, employees can quickly convert PDFs without specialized software, streamlining document processing workflows and increasing productivity.
-
Scalability for Large Volumes: The template can handle multiple PDF conversions efficiently, making it ideal for businesses dealing with large volumes of PDF documents, such as in finance, healthcare, or logistics sectors.
Technologies
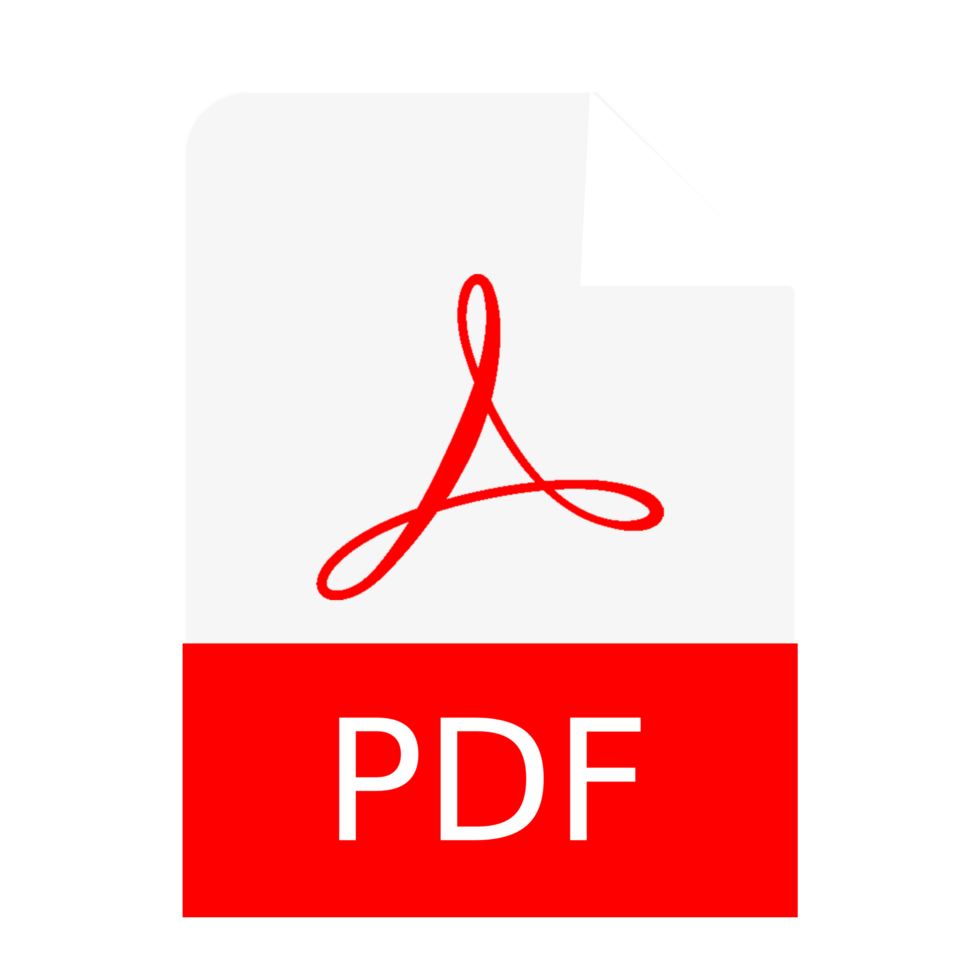