by ips
Backend Server
import logging
from fastapi import FastAPI
from fastapi.responses import RedirectResponse
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
app = FastAPI()
@app.get("/", include_in_schema=False)
def root():
return RedirectResponse(url="/docs")
@app.get("/list")
def list_entrypoint():
some_list = ["data1", "data2"]
return some_list
# Do not remove the main function while updating the app.
if __name__ == "__main__":
Backend Server
Created: | Last Updated:
Introduction to the Template
This template is designed to help you create a backend server using FastAPI. It is streamlined for building microservices or APIs with minimal frontend requirements. The template includes basic GET and POST request handlers and sets up a FastAPI application with logging.
Clicking Start with this Template
To get started, click the Start with this Template button. This will load the template into the Lazy Builder interface.
Test
Press the Test button to begin the deployment of the app. The Lazy CLI will handle the deployment process.
Entering Input
No user input is required for this template. The app will be deployed automatically.
Using the App
Once the app is deployed, you will be provided with a server link through the Lazy builder CLI. For FastAPI, you will also receive a link to the automatically generated API documentation.
- Server Link: This link can be used to interact with the API.
- Docs Link: This link will take you to the FastAPI documentation where you can explore and test the API endpoints.
Integrating the App
API Endpoints
The template provides the following API endpoints:
-
Root Endpoint
- URL:
/
- Method: GET
- Description: Redirects to the FastAPI documentation.
- Sample Request:
bash curl -X 'GET' \ 'http://<your-server-link>/' \ -H 'accept: application/json'
- Sample Response:
http HTTP/1.1 307 Temporary Redirect location: /docs
- URL:
-
List Endpoint
- URL:
/list
- Method: GET
- Description: Returns a list of sample data.
- Sample Request:
bash curl -X 'GET' \ 'http://<your-server-link>/list' \ -H 'accept: application/json'
- Sample Response:
json [ "data1", "data2" ]
- URL:
-
Custom GET Handler
- URL:
/custom-get
- Method: GET
- Description: Returns a JSON response with a message.
- Sample Request:
bash curl -X 'GET' \ 'http://<your-server-link>/custom-get' \ -H 'accept: application/json'
- Sample Response:
json { "message": "OK" }
- URL:
-
Custom POST Handler
- URL:
/custom-post
- Method: POST
- Description: Accepts a JSON payload and returns a message.
- Sample Request:
bash curl -X 'POST' \ 'http://<your-server-link>/custom-post' \ -H 'accept: application/json' \ -H 'Content-Type: application/json' \ -d '{ "field": "sample data" }'
- Sample Response:
json { "message": "Received data: sample data" }
- URL:
Adding Custom Endpoints
To add custom endpoints, you can modify the main.py
file and include additional handlers from some_get_request_handler.py
and some_post_request_handler.py
.
Example: Adding a Custom GET Endpoint
- Open
main.py
. - Import the
handle_get_endpoint
function:python from some_get_request_handler import handle_get_endpoint
- Add a new route:
python @app.get("/custom-get") def custom_get(): return handle_get_endpoint()
Example: Adding a Custom POST Endpoint
- Open
main.py
. - Import the
handle_post_endpoint
function andData
model:python from some_post_request_handler import handle_post_endpoint, Data
- Add a new route:
python @app.post("/custom-post") def custom_post(data: Data): return handle_post_endpoint(data)
By following these steps, you can extend the functionality of your FastAPI application to suit your specific needs.
Technologies
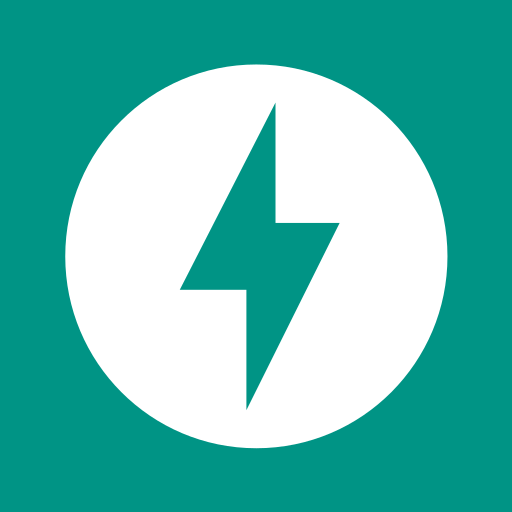
